python编写一个程序,从一个文本文件file.txt中读取文件中所存放的字符串内容,并统计其中每个单词出现的次数,以字典的形式返回结果。
时间: 2024-03-21 11:38:50 浏览: 80
好的,以下是 Python 代码:
```python
def word_count(file_name):
with open(file_name, 'r') as f:
text = f.read()
words = text.split()
word_count = {}
for word in words:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
return word_count
```
这个函数接收一个文件名作为参数,然后打开文件并读取其中的内容。接着,它将文本内容按照空格分割成一个个单词,并统计每个单词出现的次数。最后,它将结果保存在一个字典中并返回。你可以将文件名传递给这个函数,然后它会返回一个字典,其中包含每个单词出现的次数。
相关问题
编写一个程序,从一个文本文件file.txt中读取文件中所存放的字符串内容,并统计其中每个单词出现的次数,以字典的形式返回结果。
好的,这是一个Python的实现代码,你可以参考一下:
```python
def count_words(file_path):
word_count = {}
with open(file_path, 'r') as f:
for line in f:
words = line.strip().split()
for word in words:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
return word_count
```
使用方法:
```python
result = count_words('file.txt')
print(result)
```
其中,'file.txt' 是需要统计的文本文件的文件路径。函数返回的结果是一个字典,其中键表示单词,值表示该单词出现的次数。
2.编写一个程序,从一个文本文件file.txt中读取文件中所存放的字符串内容,并统计其中每个单词出现的次数,以字典的形式返回结果。
好的,以下是 Python 代码实现:
```python
def word_count(file_name):
# 打开文本文件
with open(file_name, "r") as f:
# 读取文件内容
content = f.read()
# 将字符串转换为单词列表
words = content.split()
# 统计每个单词出现的次数
word_count = {}
for word in words:
if word not in word_count:
word_count[word] = 1
else:
word_count[word] += 1
return word_count
```
使用方法:
```python
file_name = "file.txt"
result = word_count(file_name)
print(result)
```
其中,`file.txt` 是待统计的文本文件名,`result` 是统计结果,是一个字典类型的变量,键为单词,值为该单词出现的次数。
阅读全文
相关推荐
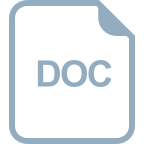
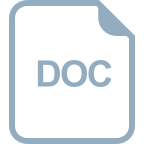
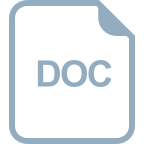













