填写程序,有两个文本文件test.1.txt和test.2.txt,各存放一行字符串,要求把这两个文件中的信息合并,按字符顺序排列,输出到一个新文件test.3.txt中,最后关闭文件
时间: 2024-02-01 15:14:49 浏览: 26
以下是Python代码实现:
```python
with open('test.1.txt', 'r') as file1, open('test.2.txt', 'r') as file2, open('test.3.txt', 'w') as file3:
# 读取文件1和文件2中的字符串并合并
merged_str = file1.readline().strip() + file2.readline().strip()
# 按字符顺序排序
sorted_str = ''.join(sorted(merged_str))
# 将排序后的字符串写入文件3中
file3.write(sorted_str)
# 关闭文件
file1.close()
file2.close()
file3.close()
```
这段代码使用了 `with` 语句来打开文件,并自动关闭文件,避免了手动关闭文件的问题。最后按照题目要求将排序后的字符串写入 `test.3.txt` 文件中,并关闭所有文件。
相关问题
编写程序,有两个文本文件test1.txt和test2.txt,各存放一行字符串 要求把这两个文件中的信息合并,按字符顺序排列,输出到一个新文件test3.txt中。最后关闭文件
好的,以下是 Python 代码实现:
```python
# 打开 test1.txt 和 test2.txt 文件,读取每行字符串
with open('test1.txt', 'r') as f1, open('test2.txt', 'r') as f2:
str1 = f1.readline().strip()
str2 = f2.readline().strip()
# 合并两个字符串并排序
merged_str = sorted(str1 + str2)
# 将结果写入 test3.txt 文件
with open('test3.txt', 'w') as f3:
f3.write(''.join(merged_str))
# 输出结果
print(f'Merged string: {"".join(merged_str)}')
```
这段代码首先使用 `with open()` 语句打开 `test1.txt` 和 `test2.txt` 文件,读取每行的字符串并去除行末的换行符。然后,将两个字符串合并为一个字符串,并使用 `sorted()` 函数对字符进行排序。最后,将排序后的结果写入 `test3.txt` 文件中并输出结果。
注意,使用 `with open()` 语句可以自动帮我们关闭文件,不需要手动调用 `close()` 方法。
编亏程序,有两个文本文件test1.txt和test2.txt,各存放一行字符串,要求把这两个文件中的信息合并,按字符顺序排列,输出到一个新文件test3.txt中。 最后关闭文任
好的,我来为您编写一个简单的Python程序来实现这个功能。请将以下代码保存为merge_files.py文件,并将test1.txt和test2.txt文件放在同一目录下,然后运行该程序即可生成test3.txt文件。
```python
# 打开test1.txt和test2.txt文件
with open('test1.txt', 'r') as file1, open('test2.txt', 'r') as file2:
# 读取文件内容
text1 = file1.read()
text2 = file2.read()
# 将两个字符串合并并按字符顺序排序
merged_text = ''.join(sorted(text1 + text2))
# 将合并后的内容写入test3.txt文件
with open('test3.txt', 'w') as file3:
file3.write(merged_text)
# 关闭文件
file1.close()
file2.close()
file3.close()
```
请注意,此程序假定输入的两个文件都只有一行字符串。如果文件中有多行字符串,程序需要进行一些修改才能正确处理。
相关推荐
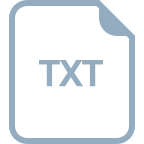
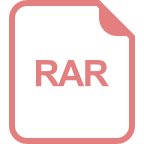
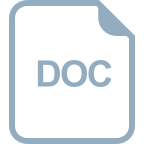












