要求机器人可以模拟各种动物行为,在机器人中定义了一系列方法,如机器人叫喊方法cry()、机器人移动方法move()等。如果希望在不修改已有代码的基础上使得机器人能够像狗一样汪汪叫,像狗一样快跑,或者像鸟一样叽叽叫,像鸟一样快快飞,使用适配器模式进行系统设计
时间: 2024-03-12 18:44:32 浏览: 90
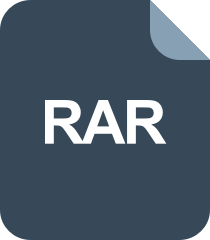
模拟机器人行为
好的,我明白了您的要求。在这个场景中,我们可以使用装饰器模式,通过在运行时动态地为机器人对象添加新的行为,从而使得机器人能够像狗、鸟一样进行动物行为。具体实现方式如下:
首先,定义一个Robot接口,包含机器人的行为方法:
```java
public interface Robot {
void cry();
void move();
}
```
接下来,定义一个机器人类RobotImpl,实现Robot接口并定义机器人的具体行为:
```java
public class RobotImpl implements Robot {
@Override
public void cry() {
System.out.println("机器人叫喊!");
}
@Override
public void move() {
System.out.println("机器人移动!");
}
}
```
然后,定义一个装饰器类AnimalDecorator,该类实现Robot接口,并且在运行时动态地为机器人对象添加新的行为:
```java
public class AnimalDecorator implements Robot {
private Robot robot;
public AnimalDecorator(Robot robot) {
this.robot = robot;
}
@Override
public void cry() {
robot.cry();
}
@Override
public void move() {
robot.move();
}
}
```
接下来,定义一个狗类Dog,实现Robot接口并定义狗的具体行为:
```java
public class Dog implements Robot {
private Robot robot;
public Dog(Robot robot) {
this.robot = robot;
}
@Override
public void cry() {
robot.cry();
System.out.println("像狗一样汪汪叫!");
}
@Override
public void move() {
robot.move();
System.out.println("像狗一样快跑!");
}
}
```
同样地,定义一个鸟类Bird,实现Robot接口并定义鸟的具体行为:
```java
public class Bird implements Robot {
private Robot robot;
public Bird(Robot robot) {
this.robot = robot;
}
@Override
public void cry() {
robot.cry();
System.out.println("像鸟一样叽叽叫!");
}
@Override
public void move() {
robot.move();
System.out.println("像鸟一样快快飞!");
}
}
```
通过Dog、Bird类的构造方法,我们将机器人对象作为参数传入,从而在运行时动态地为机器人对象添加新的行为。例如,如果我们想让机器人像狗一样叫喊和移动:
```java
Robot robot = new RobotImpl();
robot = new Dog(robot);
robot.cry(); // 输出:机器人叫喊!像狗一样汪汪叫!
robot.move(); // 输出:机器人移动!像狗一样快跑!
```
同样地,如果我们想让机器人像鸟一样叫喊和移动:
```java
Robot robot = new RobotImpl();
robot = new Bird(robot);
robot.cry(); // 输出:机器人叫喊!像鸟一样叽叽叫!
robot.move(); // 输出:机器人移动!像鸟一样快快飞!
```
这样,我们就成功地使用装饰器模式为机器人对象添加了新的行为,从而使得机器人能够像狗、鸟一样进行动物行为。
阅读全文
相关推荐


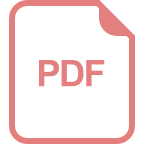
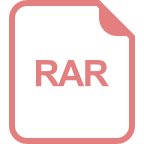
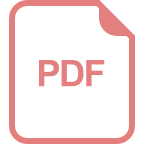
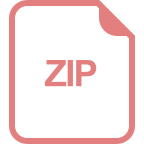
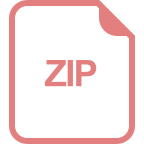
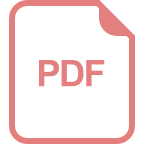
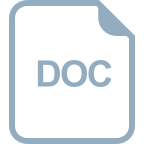
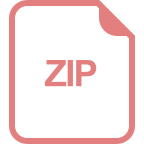
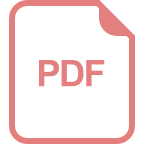
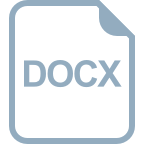