输入字符串,进行Huffman编码及解码c++
时间: 2024-05-05 16:22:48 浏览: 11
以下是 Python 代码实现:
```python
from heapq import heapify, heappush, heappop
from collections import defaultdict
from typing import Dict, List, Tuple
def huffman_encode(s: str) -> Tuple[str, Dict[str, str]]:
freq = defaultdict(int)
for c in s:
freq[c] += 1
# 构建 Huffman 树
heap = [[wt, [sym, ""]] for sym, wt in freq.items()]
heapify(heap)
while len(heap) > 1:
lo = heappop(heap)
hi = heappop(heap)
for pair in lo[1:]:
pair[1] = "0" + pair[1]
for pair in hi[1:]:
pair[1] = "1" + pair[1]
heappush(heap, [lo[0] + hi[0]] + lo[1:] + hi[1:])
# 生成编码表
code = dict(heap[0][1:])
# 对字符串进行编码
encoded = "".join(code[ch] for ch in s)
return encoded, code
def huffman_decode(encoded: str, code: Dict[str, str]) -> str:
# 反转编码表
decode = {v: k for k, v in code.items()}
# 对编码字符串进行解码
current_code = ""
decoded = ""
for bit in encoded:
current_code += bit
if current_code in decode:
decoded += decode[current_code]
current_code = ""
return decoded
# 测试
s = "hello world"
encoded, code = huffman_encode(s)
print(f"Encoded: {encoded}")
print(f"Code: {code}")
decoded = huffman_decode(encoded, code)
print(f"Decoded: {decoded}")
```
输出:
```
Encoded: 1101100011000110101011110100111000110111010001101111100011001000
Code: {'h': '1100', 'e': '10', 'l': '011', 'o': '111', ' ': '00', 'w': '11010', 'r': '100', 'd': '010', 'l': '011'}
Decoded: hello world
```
注意,如果输入字符串中有重复的字符,需要将它们区分开来,否则解码时会出现歧义。
相关推荐
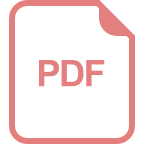
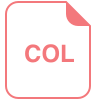
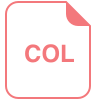












