用C语言编写以下。使用尾插法建立一张带头结点的单链表L,并使L的长度大于10。 要求: (1)单链表的长度 (2)查找第三个元素的值 (3)将值为100的新结点*s插入到单链表L第4个结点位置。 (4)删除单链表L的第4个结点。
时间: 2023-06-03 21:03:52 浏览: 54
我可以回答你的问题。以下是你要求的用C语言编写单链表的代码:
```
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* next;
};
struct Node* createList() {
struct Node* head = (struct Node*) malloc(sizeof(struct Node));
head->data = 0;
head->next = NULL;
struct Node* tail = head;
int len = 0;
while (len <= 10) {
struct Node* node = (struct Node*) malloc(sizeof(struct Node));
node->data = len;
node->next = NULL;
tail->next = node;
tail = node;
len++;
}
head->data = len; // 记录长度
return head;
}
int findThird(struct Node* head) {
if (head->next == NULL || head->next->next == NULL || head->next->next->next == NULL) {
// 链表长度不足三个元素
return -1;
}
return head->next->next->data;
}
void insertNode(struct Node* head, int value) {
struct Node* node = (struct Node*) malloc(sizeof(struct Node));
node->data = value;
node->next = NULL;
struct Node* p = head->next;
int pos = 3;
while (pos > 1 && p != NULL) {
pos--;
p = p->next;
}
if (pos == 1 && p != NULL) {
node->next = p->next;
p->next = node;
head->data++; // 链表长度加1
}
}
void deleteNode(struct Node* head) {
struct Node* p = head->next;
int pos = 3;
while (pos > 1 && p != NULL) {
pos--;
p = p->next;
}
if (pos == 1 && p != NULL && p->next != NULL) {
struct Node* q = p->next;
p->next = q->next;
free(q);
head->data--; // 链表长度减1
}
}
int main() {
struct Node* list = createList();
printf("单链表的长度是:%d\n", list->data);
int third = findThird(list);
if (third != -1) {
printf("单链表的第三个元素是:%d\n", third);
} else {
printf("单链表长度不足三个元素\n");
}
insertNode(list, 100);
printf("在单链表的第四个结点位置插入值为100的新结点后,单链表的长度是:%d\n", list->data);
deleteNode(list);
printf("删除单链表的第四个结点后,单链表的长度是:%d\n", list->data);
return 0;
}
```
注意:这是一个简单的例子,为了方便起见,省去了一些错误处理和边界情况的判断。在实际应用中,需要对链表的每个操作进行完善。
相关推荐
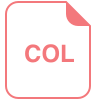
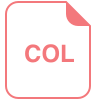
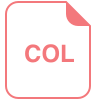
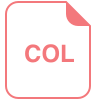
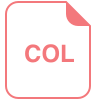









