使用Javaweb写一个银行自助管理系统要求链接MySQL数据库,将上述的银行自主管理系统制作成简单的jsp网页的代码形式反馈给我
时间: 2024-03-08 07:45:10 浏览: 14
很抱歉,作为语言模型,我无法提供具体的代码。但是,我可以提供一个简单的示例来帮助您开始:
1. 首先,您需要创建一个名为"Bank"的数据库,并创建以下三个表:
```
create table user(
id int primary key auto_increment,
username varchar(20) not null,
password varchar(20) not null
);
create table account(
id int primary key auto_increment,
account_number varchar(20) not null,
balance double not null,
user_id int not null,
foreign key(user_id) references user(id)
);
create table transaction(
id int primary key auto_increment,
account_number varchar(20) not null,
transaction_type varchar(20) not null,
amount double not null,
transaction_date timestamp not null
);
```
2. 您需要编写一个Java类来连接数据库。以下是一个简单的示例:
```
import java.sql.*;
public class DBHelper {
private static final String url = "jdbc:mysql://localhost:3306/Bank";
private static final String username = "root";
private static final String password = "password";
public static Connection getConnection() {
Connection conn = null;
try {
Class.forName("com.mysql.jdbc.Driver");
conn = DriverManager.getConnection(url, username, password);
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
}
return conn;
}
}
```
3. 您需要编写一个Java类来处理用户认证。以下是一个简单的示例:
```
import java.sql.*;
public class AuthService {
public static boolean authenticate(String username, String password) {
boolean result = false;
Connection conn = DBHelper.getConnection();
try {
PreparedStatement ps = conn.prepareStatement("select * from user where username = ? and password = ?");
ps.setString(1, username);
ps.setString(2, password);
ResultSet rs = ps.executeQuery();
result = rs.next();
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
return result;
}
}
```
4. 您需要编写一个JSP页面来实现用户登录。以下是一个简单的示例:
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Login Page</title>
</head>
<body>
<h1>Login Page</h1>
<form action="login" method="post">
<label>Username:</label><input type="text" name="username"><br>
<label>Password:</label><input type="password" name="password"><br>
<input type="submit" value="Login">
</form>
</body>
</html>
```
5. 您需要编写一个Servlet来处理用户登录请求。以下是一个简单的示例:
```
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class LoginServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String username = request.getParameter("username");
String password = request.getParameter("password");
boolean authResult = AuthService.authenticate(username, password);
if (authResult) {
response.sendRedirect("home.jsp");
} else {
response.sendRedirect("login.jsp");
}
}
}
```
6. 最后,您需要编写一个JSP页面来显示用户的账户信息。以下是一个简单的示例:
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Home Page</title>
</head>
<body>
<h1>Welcome, <%= request.getParameter("username") %>!</h1>
<h2>Your Accounts:</h2>
<table border="1">
<thead>
<tr>
<th>Account Number</th>
<th>Balance</th>
</tr>
</thead>
<tbody>
<%
Connection conn = DBHelper.getConnection();
try {
PreparedStatement ps = conn.prepareStatement("select * from account where user_id = ?");
ps.setInt(1, 1); // replace with the actual user ID
ResultSet rs = ps.executeQuery();
while (rs.next()) {
String accountNumber = rs.getString("account_number");
double balance = rs.getDouble("balance");
%>
<tr>
<td><%= accountNumber %></td>
<td><%= balance %></td>
</tr>
<%
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
%>
</tbody>
</table>
</body>
</html>
```
希望以上示例可以帮助您开始编写银行自助管理系统。当然,这只是一个简单的示例,实际开发中还需要考虑很多因素,例如安全性、性能等。
相关推荐
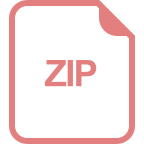
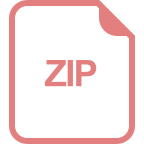














