ld3320与stm32串口连接
时间: 2023-05-28 10:05:16 浏览: 290
要将LD3320与STM32串口连接,首先需要了解LD3320和STM32的串口通信协议。LD3320是一款语音识别模块,可以通过串口与控制器进行通信,实现语音识别、录音和播放等功能。而STM32是一款微控制器芯片,也支持串口通信。
以下是LD3320和STM32串口连接步骤:
1. 确定LD3320的串口通信参数,包括波特率、数据位、校验位和停止位等。
2. 在STM32中配置串口参数,使其与LD3320的参数相匹配。可以使用STM32的串口配置工具进行配置,也可以在代码中手动设置。
3. 连接LD3320的串口信号线(TX、RX)到STM32的对应引脚。
4. 在STM32代码中使用串口通信函数,向LD3320发送指令并接收响应。可以使用HAL库提供的串口通信函数,也可以自行编写。
5. 根据LD3320和STM32的串口通信协议,解析LD3320返回的数据并进行相应的处理。
需要注意的是,LD3320和STM32的串口通信协议可能因厂商和产品型号而异,需要仔细查看相关文档并进行适当的调整。同时,在连接过程中也要注意保持信号线的正确连接和电气参数的匹配,确保稳定可靠的通信。
相关问题
ld3320与stm32串口通信
LD3320是一个语音识别模块,可以通过串口与STM32进行通信。下面是基本的串口通信代码示例:
```c
#include "stm32f10x.h"
/* 定义串口发送函数 */
void USART_SendString(USART_TypeDef* USARTx, char* s)
{
while(*s)
{
while(USART_GetFlagStatus(USARTx, USART_FLAG_TC) == RESET);
USART_SendData(USARTx, *s++);
}
}
int main(void)
{
/* 初始化串口 */
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1 | RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
USART_InitStructure.USART_BaudRate = 115200;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Tx | USART_Mode_Rx;
USART_Init(USART1, &USART_InitStructure);
USART_Cmd(USART1, ENABLE);
while(1)
{
/* 发送命令给LD3320,例如"LD3320,START" */
char command[] = "LD3320,START";
USART_SendString(USART1, command);
/* 接收LD3320返回的数据 */
char receiveBuffer[100];
int i = 0;
while(USART_GetFlagStatus(USART1, USART_FLAG_RXNE) == RESET);
while(USART_GetFlagStatus(USART1, USART_FLAG_RXNE) != RESET)
{
receiveBuffer[i] = USART_ReceiveData(USART1);
i++;
}
/* 处理接收到的数据 */
// ...
}
}
```
在上面的示例中,我们使用USART1作为串口,并将PA9和PA10引脚分别连接到LD3320的TX和RX引脚。在主循环中,我们发送命令给LD3320,然后等待LD3320返回数据,并将接收到的数据存储在receiveBuffer数组中。接收到的数据可以进行相应的处理。
ld3320与stm32串口通讯
LD3320是一款语音识别模块,可以通过串口与STM32进行通讯。下面是一个简单的示例代码,可以帮助你了解如何实现LD3320与STM32的串口通讯:
```c
#include "stm32f10x.h"
void USART1_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_USART1, ENABLE);
// 配置USART1的GPIO
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9; // USART1_TX
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10; // USART1_RX
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 配置USART1
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Tx | USART_Mode_Rx;
USART_Init(USART1, &USART_InitStructure);
USART_Cmd(USART1, ENABLE);
}
void USART1_SendByte(uint8_t byte)
{
while (USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET);
USART_SendData(USART1, byte);
}
uint8_t USART1_ReceiveByte(void)
{
while (USART_GetFlagStatus(USART1, USART_FLAG_RXNE) == RESET);
return USART_ReceiveData(USART1);
}
int main(void)
{
USART1_Init();
// 向LD3320发送命令
USART1_SendByte(0xAA);
USART1_SendByte(0x00);
USART1_SendByte(0x03);
USART1_SendByte(0x02);
USART1_SendByte(0x01);
USART1_SendByte(0x00);
// 接收LD3320的响应
uint8_t response[6];
for (int i = 0; i < 6; i++)
{
response[i] = USART1_ReceiveByte();
}
while (1)
{
// 你的代码
}
}
```
在上面的代码中,我们首先初始化了USART1,并向LD3320发送了一个命令。然后,我们通过USART1接收LD3320的响应,并将其保存在response数组中。最后,我们进入一个无限循环,可以在其中编写自己的代码。
需要注意的是,LD3320的通讯协议非常复杂,具体的使用方法需要参考其官方文档。这里只是给出了一个简单的示例,供参考。
阅读全文
相关推荐
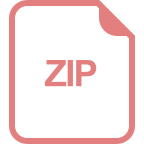
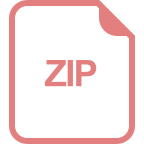
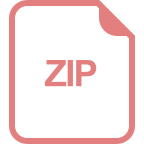
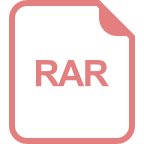
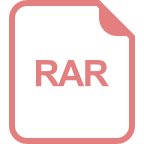
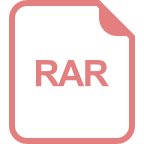






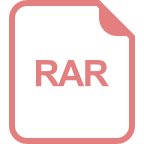
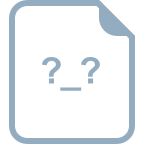
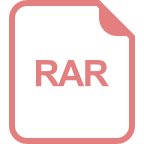