what is the lambda function in CPP
时间: 2024-02-21 12:00:02 浏览: 57
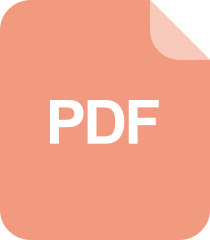
有效使用Lambda表达式和std::function
In C++, a lambda function is an anonymous function that can be defined inline with the rest of your code. It allows you to create a function object on the fly, without having to define a separate function. Lambda functions can capture variables from the surrounding context and can be passed around like any other object. Here's an example of a lambda function in C++:
```
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> nums{3, 6, 2, 7, 1, 9};
// Sort the vector in descending order using a lambda function
std::sort(nums.begin(), nums.end(), [](int a, int b) {
return a > b;
});
// Print the sorted vector
for (int num : nums) {
std::cout << num << " ";
}
return 0;
}
```
In this example, the lambda function is used as the third argument to the `std::sort` algorithm. The function takes two integers as arguments and returns `true` if the first argument is greater than the second argument, indicating that the vector should be sorted in descending order.
阅读全文
相关推荐
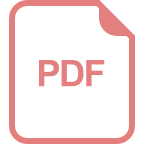
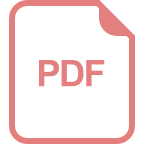

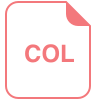
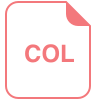
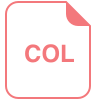
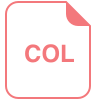
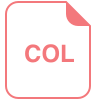
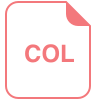
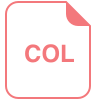
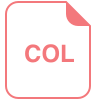
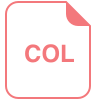
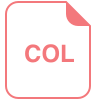
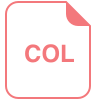
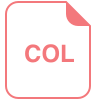
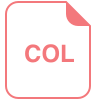
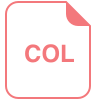