python多线程 线程隔离
时间: 2023-08-27 11:06:32 浏览: 180
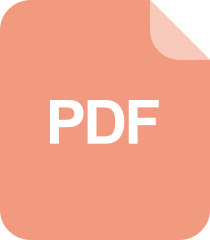
python多线程与多进程及其区别详解
在 Python 中,可以使用 `threading` 模块来实现多线程编程。多线程可以帮助我们同时执行多个任务,提高程序的性能和效率。
线程隔离是指在多线程编程中,每个线程都有自己独立的数据空间,不会被其他线程访问和修改。这样可以防止多个线程之间的数据竞争和冲突。
Python 中的线程隔离可以通过以下方式实现:
1. 使用 threading.local() 创建一个本地线程变量。这样每个线程都可以独立地使用和修改该变量,而不会影响其他线程。例如:
```python
import threading
# 创建本地线程变量
local_data = threading.local()
# 在每个线程中设置和访问变量
def thread_func():
local_data.value = 10
print(local_data.value)
# 创建多个线程并启动
threads = []
for _ in range(5):
t = threading.Thread(target=thread_func)
threads.append(t)
t.start()
# 等待所有线程完成
for t in threads:
t.join()
```
上述代码中,每个线程都能够独立地设置和访问 `local_data.value` 变量,互不干扰。
2. 使用 threading.Lock() 或 threading.RLock() 来保护共享资源的访问。这样可以确保同一时间只有一个线程在访问共享资源,避免数据竞争的问题。
```python
import threading
# 创建共享资源和锁
shared_resource = 0
lock = threading.Lock()
# 在每个线程中访问共享资源
def thread_func():
global shared_resource
with lock:
shared_resource += 1
print(shared_resource)
# 创建多个线程并启动
threads = []
for _ in range(5):
t = threading.Thread(target=thread_func)
threads.append(t)
t.start()
# 等待所有线程完成
for t in threads:
t.join()
```
上述代码中,通过使用锁 `lock` 来确保同一时间只有一个线程在访问 `shared_resource` 共享资源,避免了数据竞争的问题。
请注意,在 Python 中,由于全局解释器锁 (GIL) 的存在,多线程并不能真正实现并行执行,而是通过在不同的时间片轮换执行不同的线程来模拟并发。如果需要利用多核 CPU 实现真正的并行计算,可以考虑使用多进程编程。
阅读全文
相关推荐
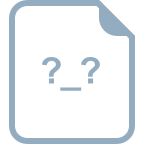
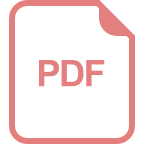















