friend MyVector<T> operator*(size_t scalar1,const MyVector<T>& scalar2);怎么把他变成模板函数
时间: 2024-10-15 13:26:12 浏览: 12
`friend MyVector<T> operator*(size_t scalar1, const MyVector<T>& scalar2);` 这行代码声明了一个友元函数,它接受一个 `size_t` 类型的 `scalar1` 和一个 `MyVector<T>` 类型的引用 `scalar2`,并返回一个同类型的 `MyVector<T>`。如果你想将其转换为一个模板函数,可以这样做:
```cpp
template<typename U>
friend MyVector<U> operator*(size_t scalar1, const MyVector<U>& scalar2);
```
这里,`U` 是一个类型参数,表示友元函数可以处理任何类型的 `MyVector`,而不仅仅是模板实例化时指定的类型 `T`。这意味着无论何时调用这个友元函数,它都可以用于任何 `MyVector` 类型,只要传递的 `MyVector` 的类型与 `U` 匹配即可。
例如,如果你有一个 `MyVector<int>` 对象和一个 `MyVector<double>` 对象,这个模板友元函数就可以对两者都进行乘法操作,因为它们都是 `MyVector` 的实例。
请注意,虽然这个友元函数现在可以处理多种类型的 `MyVector`,但在实际使用时,还需要确保模板函数内部的逻辑也支持通用的类型 `U`。
相关问题
[Warning] friend declaration 'MyVector<T> operator*(const T&, const MyVector<T>&)' declares a non-template function [-Wnon-template-friend]
这个警告提示是在C++中遇到的一个问题,表示你在声明友元函数 `MyVector<T> operator*(const T&, const MyVector<T>&)` 时,并没有将其指定为模板函数。然而,由于 `T` 是一个类型参数,通常在这种涉及泛型操作符重载的情况下,你需要明确地声明它是一个模板函数。
如果你的意图是为所有类型的 `MyVector` 实例提供通用的乘法操作,你应该将 `operator*` 定义为模板函数,如下所示:
```cpp
template<typename T>
friend MyVector<T> operator*(const T& scalar, const MyVector<T>& vector);
```
或者如果需要支持两个 `MyVector` 的元素间操作,则应为模板函数,并接受两个 `MyVector<T>` 类型的参数:
```cpp
template<typename T>
class MyVector {
public:
template<typename U>
friend MyVector<U> operator*(const MyVector<T>& first, const MyVector<U>& second); // 或者仅限于同类型的操作
// 其他函数...
};
```
这个警告通常意味着你需要修改你的友元函数声明或定义,以适应模板函数的要求。
请用c++完成:#include <iostream> #include <vector> #include <algorithm> using namespace std; template<class T> class MyVector { public: void push_back(T x) { vec.push_back(x); } void sort() { std::sort(vec.begin(), vec.end()); } void output1() { for (int i = 0; i < vec.size(); i++) { cout << vec[i] << " "; } cout << endl; } void output2() { for (auto it = vec.begin(); it != vec.end(); it++) { cout << *it << " "; } cout << endl; } void output3() { for_each(vec.begin(), vec.end(), [](T x) { cout << x << " "; }); cout << endl; } private: vector<T> vec; }; int main() { int x; MyVector<int> v; while (cin >> x && x != 0) { v.push_back(x); } v.sort(); v.output1(); v.output2(); v.output3(); return 0; }
以下是 C++ 代码:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
template<class T>
class MyVector {
public:
void push_back(T x) {
vec.push_back(x);
}
void sort() {
std::sort(vec.begin(), vec.end());
}
void output1() {
for (int i = 0; i < vec.size(); i++) {
cout << vec[i] << " ";
}
cout << endl;
}
void output2() {
for (auto it = vec.begin(); it != vec.end(); it++) {
cout << *it << " ";
}
cout << endl;
}
void output3() {
for_each(vec.begin(), vec.end(), [](T x) { cout << x << " "; });
cout << endl;
}
private:
vector<T> vec;
};
int main() {
int x;
MyVector<int> v;
while (cin >> x && x != 0) {
v.push_back(x);
}
v.sort();
v.output1();
v.output2();
v.output3();
return 0;
}
```
该程序定义了一个模板类 MyVector,其中包含了 push_back,sort,output1,output2 和 output3 函数。在主函数中,首先创建了一个 MyVector 类型的对象 v,并循环读入整数并插入到 v 中。然后,对 v 进行排序,并输出三种不同的方式:output1 输出方式使用下标访问 vector 中的元素,output2 输出方式使用迭代器遍历 vector 中的元素,output3 输出方式使用 for_each 函数遍历 vector 中的元素。
阅读全文
相关推荐
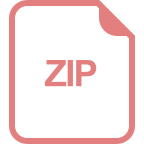
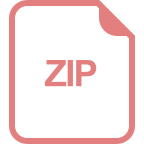













