n=int(input()) a=list(map(int,input().split(' '))) res=1e6+10 for x in a: if x%2==0: res=min(res,x) print(res)改成C++代码
时间: 2024-12-20 10:29:09 浏览: 4
这段Python代码的功能是从用户输入的一串整数中找出最小的偶数,如果不存在偶数则输出默认值1e6+10。以下是将这个功能转换成C++代码:
```cpp
#include <iostream>
#include <vector>
#include <climits>
int main() {
int n;
std::cin >> n;
std::vector<int> a(n);
for (int i = 0; i < n; ++i) {
std::cin >> a[i];
}
int res = INT_MAX;
for (int x : a) {
if (x % 2 == 0) {
res = std::min(res, x);
}
}
if (res != INT_MAX) {
std::cout << res << "\n";
} else {
std::cout << "1e6+10\n"; // 输出默认值
}
return 0;
}
```
在这个C++代码中,我们使用了`std::cin`读取用户输入,`std::vector`存储整数数组,`INT_MAX`作为最大值的预设,然后通过范围-based for循环遍历数组查找偶数。最后根据结果打印或输出默认值。
相关问题
n=int(input()) a=list(map(int,input().split(' '))) res=1e6+10 for x in a: if x%2==0: res=min(res,x) print(res)转c++
这段Python代码的主要功能是从用户输入的一串整数中找出最小的偶数,如果所有数都是奇数则返回1000001。下面是将该代码转换成C++的版本:
```cpp
#include <iostream>
#include <vector>
#include <climits>
int main() {
int n;
std::cin >> n;
std::vector<int> a(n);
for (int i = 0; i < n; ++i) {
std::cin >> a[i];
}
int res = INT_MAX; // 使用INT_MAX代替1e6+10
for (int x : a) { // 更简洁的迭代方式
if (x % 2 == 0) {
res = std::min(res, x);
}
}
std::cout << (res == INT_MAX ? -1 : res) << std::endl; // 输出结果可能是负数,所以这里判断一下
return 0;
}
```
n=int(input()) a=list(map(int,input().split(' '))) res=1e6+10 for x in a: if x%2==0: res=min(res,x) print(res)用C语言
这段Python代码是用来计算列表`a`中所有偶数元素的最小值。首先通过`input()`获取整数n,然后输入n个空格分隔的整数到列表a。接下来,初始化变量`res`为一个非常大的数,遍历列表`a`,如果遇到的数字x是偶数(`x % 2 == 0`),就更新`res`为其当前值和x中的较小值。最后输出这个最小的偶数值。
以下是等效的C语言代码:
```c
#include <stdio.h>
#include <limits.h>
int main() {
int n;
scanf("%d", &n);
int a[n];
for (int i = 0; i < n; i++) {
scanf("%d", &a[i]);
}
int res = INT_MAX; // 初始化为最大整数
for (int x : a) { // 使用C99的范围基础for循环代替map
if (x % 2 == 0) {
res = min(res, x);
}
}
printf("%d\n", res);
return 0;
}
```
注意:这里使用了`INT_MAX`来代替`1e6 + 10`,表示非常大。C标准库中的`<limits.h>`定义了`INT_MAX`常量。
阅读全文
相关推荐
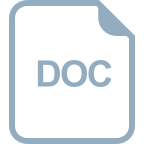
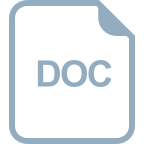
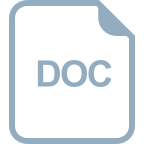
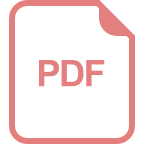
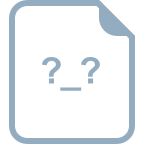
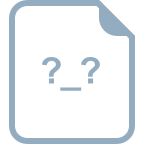
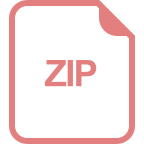
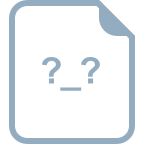
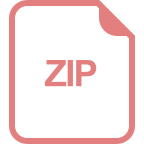
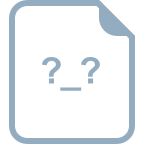
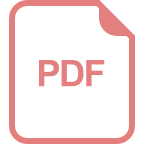
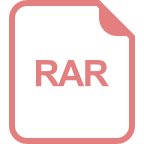
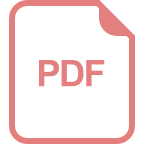
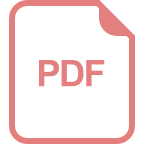
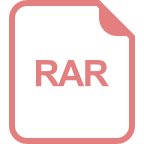
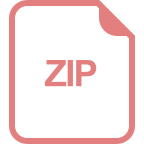