Java使用随机数模拟剪刀、石头、布游戏,采用五局三胜制。(do...while语句) 提示:使用Math.randorm()生成随机数。
时间: 2024-09-29 07:11:15 浏览: 41
在Java中,我们可以使用`Math.random()`函数生成0到1之间的随机数来模拟剪刀、石头、布游戏。以下是一个使用do...while循环和五局三胜制的简单示例:
```java
import java.util.Random;
public class RockPaperScissors {
enum GameResult { Player1Win, Tie, Player2Win }
public static void main(String[] args) {
Random random = new Random();
int playerScore = 0;
int computerScore = 0;
int gameCount = 0;
do {
gameCount++;
System.out.printf("第%d局:\n", gameCount);
// 电脑随机选择(0代表石头,1代表剪刀,2代表布)
int computerChoice = random.nextInt(3);
// 用户选择
System.out.println("请输入你的选择(0-石头,1-剪刀,2-布):");
int userChoice = getUserChoice();
// 判断胜负
GameResult result = playGame(userChoice, computerChoice);
switch (result) {
case Player1Win:
System.out.println("玩家赢了!");
playerScore++;
break;
case Tie:
System.out.println("平局!");
break;
case Player2Win:
System.out.println("电脑赢了!");
computerScore++;
break;
}
// 输出当前分数
System.out.println("玩家得分:{},电脑得分:{}", playerScore, computerScore);
// 检查是否达到胜利条件
if (playerScore >= 3 || computerScore >= 3) {
break;
}
} while (gameCount < 5); // 只进行五局
// 游戏结束,显示最终结果
System.out.println("\n游戏结束,胜者是:");
if (playerScore > computerScore) {
System.out.println("玩家");
} else if (computerScore > playerScore) {
System.out.println("电脑");
} else {
System.out.println("平局");
}
}
private static int getUserChoice() {
// 省略错误处理,假设用户总是输入有效选项
return ...; // 实际应用中应添加用户输入验证
}
private static GameResult playGame(int userChoice, int computerChoice) {
// 石头砸剪刀,剪刀剪布,布包石头
switch ((userChoice + computerChoice) % 3) {
case 0: return GameResult.Player1Win;
case 1: return GameResult.Player2Win;
default: return GameResult.Tie;
}
}
}
阅读全文
相关推荐
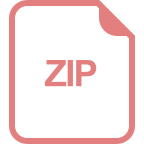
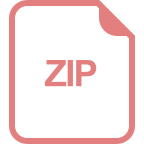
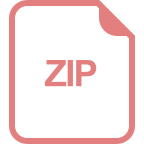
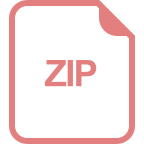
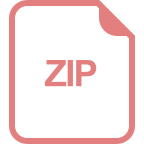
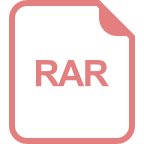
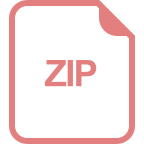
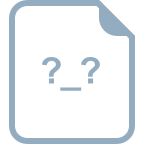
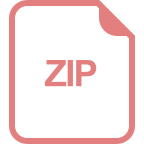
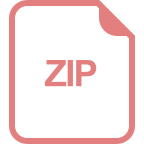
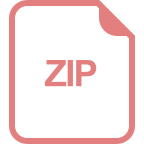
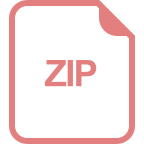
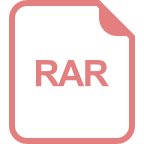
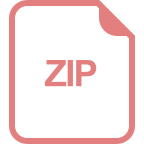
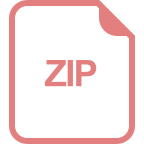
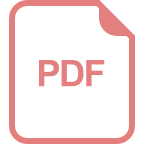
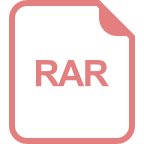
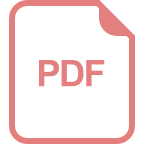