编写Java应用程序,实现稀疏矩阵的加法和乘法运算,其中稀疏矩阵是指矩阵中的大部分元素的值为0。用户在命令行输入矩阵时矩阵的大小可能有错,因此需要使用异常处理。
时间: 2024-12-16 21:28:37 浏览: 1
在Java中,可以使用`java.util.Map`数据结构以及自定义`SparseMatrix`类来实现稀疏矩阵的加法和乘法。首先,我们需要定义一个`SparseMatrix`类,它通常包含两个内部数据结构:一个是键值对数组表示非零元素的位置和值,另一个用于存储行数和列数。
```java
import java.util.HashMap;
import java.util.Map;
class SparseMatrix {
private int rows, cols;
private Map<Integer, Map<Integer, Integer>> matrix;
// 构造函数、getters和setters省略
public void add(SparseMatrix other) throws Exception {
if (rows != other.cols || cols != other.rows) {
throw new IllegalArgumentException("Matrices can't be added because of incompatible dimensions");
}
for (Map.Entry<Integer, Map<Integer, Integer>> row : other.matrix.entrySet()) {
for (Map.Entry<Integer, Integer> entry : row.getValue().entrySet()) {
put(entry.getKey(), row.getKey(), entry.getValue());
}
}
}
public void multiply(SparseMatrix other) throws Exception {
// 稀疏矩阵相乘的算法会更复杂,这里仅给出基本思路
if (cols != other.rows) {
throw new IllegalArgumentException("Incompatible dimensions for multiplication");
}
Map<Integer, Map<Integer, Integer>> result = new HashMap<>();
for (int i = 0; i < rows; i++) {
for (int j = 0; j < other.cols; j++) {
int value = 0;
for (Map.Entry<Integer, Integer> entry : getRow(i).entrySet()) {
int k = getCol(entry.getKey());
if (other.matrix.containsKey(k) && other.getRow(k).containsKey(j)) {
value += get(i, k) * other.get(k, j);
}
}
if (value != 0) {
result.put(i, putIfAbsent(j, value));
}
}
}
}
// 其他辅助方法如getRow, getCol, put, putIfAbsent等省略
}
```
用户可以在命令行通过读取用户的输入并解析来构建矩阵,同时使用try-catch块捕获可能出现的异常:
```java
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter the number of rows and columns:");
try {
int rows = scanner.nextInt();
int cols = scanner.nextInt();
SparseMatrix first = parseInput(scanner, rows, cols);
System.out.println("Enter second matrix...");
SparseMatrix second = parseInput(scanner, rows, cols);
SparseMatrix result;
try {
result = first.add(second);
// 或者
result = first.multiply(second);
} catch (Exception e) {
System.err.println(e.getMessage());
return;
}
// 打印结果矩阵
result.print();
} catch (Exception e) {
System.err.println("Invalid input: " + e.getMessage());
}
scanner.close();
}
private static SparseMatrix parseInput(Scanner scanner, int rows, int cols) {
// 实现从用户输入解析稀疏矩阵的代码
}
```
在上述代码中,`parseInput`方法用于将用户输入转换成稀疏矩阵,如果输入不符合规范,例如非法的矩阵尺寸,该方法将抛出异常。当矩阵加法或乘法完成后,用户可能会看到错误消息或正常打印的结果。
阅读全文
相关推荐
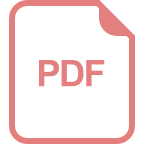
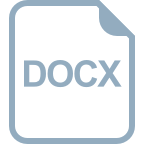
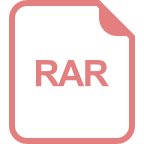
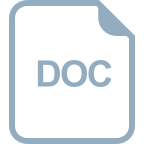
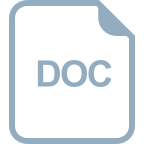
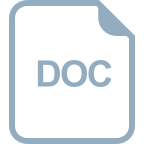
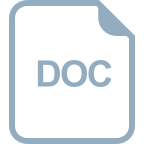
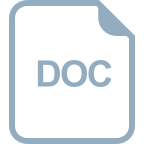
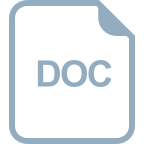
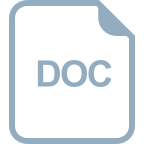



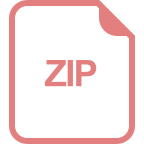
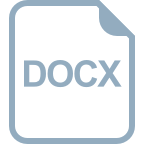