1.请编程实现一个对顺序表进行基本操作的系统,主要包括表的创建、输入、查询、取值、插入、删除和输出等操作。
时间: 2023-12-20 13:04:59 浏览: 67
以下是一个基本的顺序表操作系统的 Python 代码实现:
```python
class SeqList:
def __init__(self, max_size):
self.max_size = max_size
self.data = [None] * max_size
self.length = 0
def is_empty(self):
return self.length == 0
def is_full(self):
return self.length == self.max_size
def insert(self, index, value):
if self.is_full():
print("Error: SeqList is full!")
return False
if index < 0 or index > self.length:
print("Error: Invalid index!")
return False
for i in range(self.length, index, -1):
self.data[i] = self.data[i-1]
self.data[index] = value
self.length += 1
return True
def delete(self, index):
if self.is_empty():
print("Error: SeqList is empty!")
return False
if index < 0 or index >= self.length:
print("Error: Invalid index!")
return False
for i in range(index, self.length-1):
self.data[i] = self.data[i+1]
self.data[self.length-1] = None
self.length -= 1
return True
def get_value(self, index):
if index < 0 or index >= self.length:
print("Error: Invalid index!")
return None
return self.data[index]
def search(self, value):
for i in range(self.length):
if self.data[i] == value:
return i
return -1
def input_data(self):
for i in range(self.max_size):
value = int(input("Please input value for index %d: " % i))
if value == -1:
break
self.data[i] = value
self.length += 1
def output_data(self):
print("SeqList: ", end="")
for i in range(self.length):
print(self.data[i], end=" ")
print()
max_size = 10
seq_list = SeqList(max_size)
while True:
print("1. Create SeqList")
print("2. Insert element")
print("3. Delete element")
print("4. Get value of an element")
print("5. Search element")
print("6. Output SeqList")
print("0. Exit")
choice = int(input("Please input your choice: "))
if choice == 0:
break
elif choice == 1:
seq_list.input_data()
elif choice == 2:
index = int(input("Please input the index to insert: "))
value = int(input("Please input the value to insert: "))
seq_list.insert(index, value)
elif choice == 3:
index = int(input("Please input the index to delete: "))
seq_list.delete(index)
elif choice == 4:
index = int(input("Please input the index to get value: "))
value = seq_list.get_value(index)
if value is not None:
print("Value of index %d is: %d" % (index, value))
elif choice == 5:
value = int(input("Please input the value to search: "))
index = seq_list.search(value)
if index != -1:
print("Value %d is at index %d" % (value, index))
else:
print("Value %d not found!" % value)
elif choice == 6:
seq_list.output_data()
else:
print("Invalid choice, please try again!")
```
运行上述代码,可以实现一个简单的顺序表操作系统,支持创建、插入、删除、取值、查询和输出等基本操作。通过菜单式的交互界面,用户可以方便地进行各种操作。
阅读全文
相关推荐
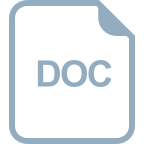
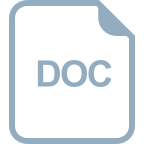
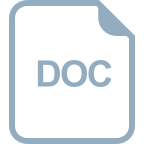














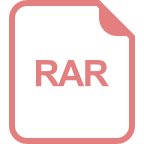