写出stm32f103c6t6用LCD1602显示数字的具体程序代码
时间: 2023-03-06 12:17:22 浏览: 123
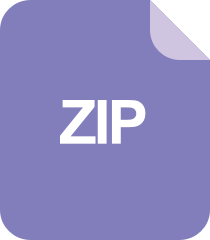
基于stm32 的LCD1602驱动代码
要写出STM32F103C6T6用LCD1602显示数字的程序代码,需要先定义一些需要用到的函数,例如:用来初始化LCD1602的函数,用来写入数据的函数,用来设置显示模式的函数等等,然后再根据需求编写相应的程序代码。具体代码如下:// 初始化LCD1602
void LCD1602_Init(void)
{
// 其他初始化步骤
}// 写入数据
void LCD1602_WriteData(uint8_t data)
{
// 其他写入数据步骤
}// 设置显示模式
void LCD1602_SetMode(uint8_t mode)
{
// 其他设置模式步骤
}// 显示数字
void LCD1602_DisplayNumber(uint16_t number)
{
// 其他显示数字步骤
}// 主函数
int main(void)
{
LCD1602_Init(); // 初始化LCD1602
LCD1602_SetMode(0x0C); // 设置显示模式
LCD1602_DisplayNumber(12345); // 显示数字
while(1); // 死循环
}
阅读全文
相关推荐









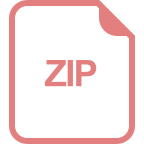