不适用pcl库将点云数据转换成二进制数据
时间: 2023-07-30 09:06:23 浏览: 178
好的,如果您不想使用PCL库,可以手动将点云数据转换为二进制数据。以下是一个简单的示例代码,用于将点云数据转换为二进制数据:
```
#include <iostream>
#include <fstream>
#include <vector>
struct Point
{
float x;
float y;
float z;
};
int main()
{
// Read point cloud data
std::vector<Point> point_cloud;
std::ifstream infile("input.txt");
float x, y, z;
while (infile >> x >> y >> z)
{
Point p;
p.x = x;
p.y = y;
p.z = z;
point_cloud.push_back(p);
}
infile.close();
// Convert to binary data
std::vector<char> binary_data;
for (auto& p : point_cloud)
{
char* ptr = reinterpret_cast<char*>(&p);
for (int i = 0; i < sizeof(Point); i++)
{
binary_data.push_back(*ptr++);
}
}
// Save binary data to file
std::ofstream outfile("output.bin", std::ios::binary);
outfile.write(binary_data.data(), binary_data.size());
outfile.close();
return 0;
}
```
在上面的代码中,我们首先读取点云数据(在这里使用文本文件),然后将其存储在一个`std::vector<Point>`对象中。然后,我们循环遍历点云数据,将每个点转换为一个二进制数据,并将其存储在一个`std::vector<char>`对象中。
为了将每个点转换为二进制数据,我们使用了`reinterpret_cast`来将`Point`结构体转换为一个`char`数组指针,然后将该数组指针中的数据一个一个地写入到`std::vector<char>`对象中。
最后,我们将二进制数据保存到文件中,以备将来使用。
请注意,这只是一个简单的示例代码,您需要根据实际情况进行修改以满足您的需求。
阅读全文
相关推荐
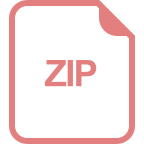
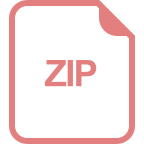
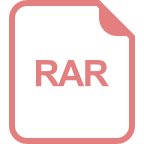
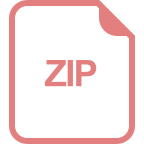
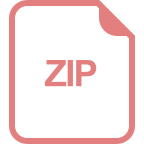
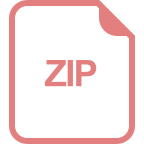
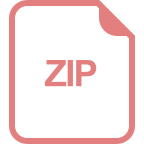
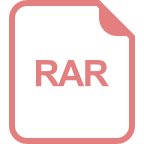
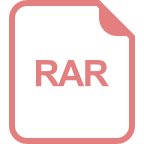
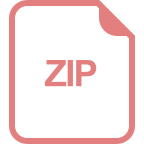
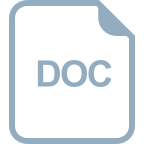
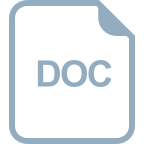
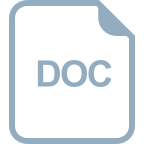



