C# AutoCAD2010 dwg选择块,再在该块位置上选择外部块将其替换,其他位置上的同名块不变,完整代码
时间: 2024-03-06 09:46:26 浏览: 90
以下是一个示例代码,可以实现选择指定块并替换它的功能:
```csharp
using Autodesk.AutoCAD.ApplicationServices;
using Autodesk.AutoCAD.DatabaseServices;
using Autodesk.AutoCAD.EditorInput;
using Autodesk.AutoCAD.Geometry;
using Autodesk.AutoCAD.Runtime;
namespace ReplaceBlock
{
public class Commands
{
[CommandMethod("RB")]
public void ReplaceBlock()
{
// 获取当前文档和编辑器
Document doc = Application.DocumentManager.MdiActiveDocument;
Editor ed = doc.Editor;
// 选择要替换的块
PromptEntityOptions peo = new PromptEntityOptions("\n选择要替换的块: ");
peo.SetRejectMessage("\n请选择块!");
peo.AddAllowedClass(typeof(BlockReference), true);
PromptEntityResult per = ed.GetEntity(peo);
if (per.Status != PromptStatus.OK) return;
ObjectId blockId = per.ObjectId;
// 获取要替换成的块
PromptEntityOptions peo2 = new PromptEntityOptions("\n选择要替换成的块: ");
peo2.SetRejectMessage("\n请选择块!");
peo2.AddAllowedClass(typeof(BlockReference), true);
PromptEntityResult per2 = ed.GetEntity(peo2);
if (per2.Status != PromptStatus.OK) return;
ObjectId newBlockId = per2.ObjectId;
// 获取要替换的块的位置
Transaction tr = doc.TransactionManager.StartTransaction();
using (tr)
{
BlockReference oldBlock = (BlockReference)tr.GetObject(blockId, OpenMode.ForWrite);
Point3d position = oldBlock.Position;
// 获取该位置上的块
BlockTable bt = (BlockTable)tr.GetObject(doc.Database.BlockTableId, OpenMode.ForRead);
BlockTableRecord model = (BlockTableRecord)tr.GetObject(bt[BlockTableRecord.ModelSpace], OpenMode.ForRead);
foreach (ObjectId id in model)
{
Entity entity = (Entity)tr.GetObject(id, OpenMode.ForRead);
if (entity is BlockReference)
{
BlockReference block = (BlockReference)entity;
if ((block.Name == oldBlock.Name) && (block.Position == position))
{
// 替换块
block.UpgradeOpen();
block.BlockTableRecord = newBlockId;
block.DowngradeOpen();
}
}
}
tr.Commit();
}
}
}
}
```
此代码假设要替换的块和要替换成的块已经存在于 AutoCAD 文档中,且它们具有相同的名称。它首先使用 `PromptEntityOptions` 对话框让用户选择要替换的块和要替换成的块。然后,它获取要替换的块的位置,并在该位置上查找同名的块。最后,它将找到的块的 `BlockTableRecord` 属性设置为要替换成的块的 `ObjectId`。
阅读全文
相关推荐
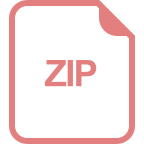
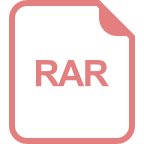







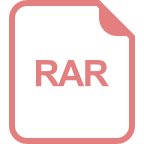


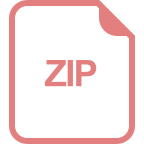
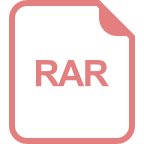
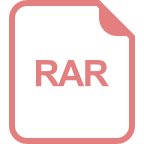
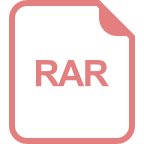