输出int,float,double,char的大小用Python写
时间: 2024-05-20 21:17:27 浏览: 11
int_size = sizeof(int)
float_size = sizeof(float)
double_size = sizeof(double)
char_size = sizeof(char)
print("Size of int: ", int_size)
print("Size of float: ", float_size)
print("Size of double: ", double_size)
print("Size of char: ", char_size)
相关问题
使用python写一个词法分析器200行代码
以下是一个简单的词法分析器代码示例,仅供参考,可能不够完善:
```python
import re
keywords = ['if', 'else', 'while', 'for', 'int', 'float', 'double', 'char', 'void']
operators = ['+', '-', '*', '/', '=', '>', '<', '>=', '<=', '==', '!=']
delimiters = [';', ',', '(', ')', '{', '}']
def tokenizer(code):
tokens = []
i = 0
while i < len(code):
if code[i].isspace():
i += 1
elif code[i].isalpha():
match = re.match(r'^[a-zA-Z]\w*', code[i:])
word = match.group()
if word in keywords:
tokens.append(('keyword', word))
else:
tokens.append(('identifier', word))
i += len(word)
elif code[i].isdigit():
match = re.match(r'^\d+', code[i:])
num = match.group()
tokens.append(('number', num))
i += len(num)
elif code[i] in operators:
if code[i:i+2] in operators:
tokens.append(('operator', code[i:i+2]))
i += 2
else:
tokens.append(('operator', code[i]))
i += 1
elif code[i] in delimiters:
tokens.append(('delimiter', code[i]))
i += 1
else:
i += 1
return tokens
code = """
int main() {
int a = 1;
if (a == 1) {
printf("Hello, world!");
}
return 0;
}
"""
tokens = tokenizer(code)
for token in tokens:
print(token)
```
这个词法分析器使用正则表达式进行匹配,将代码分解为多个token,每个token都是一个元组,第一个元素是token类型,第二个元素是token的值。这个词法分析器仅支持简单的代码,可能不够完善,仅供参考。
void GetDeviceInfo(const char* pDeviceMac, SDeviceInfo& rDeviceInfo) unsigned int GetNumberOfAttribute(const char* pDeviceMac) python调用
要在 Python 中调用 C 函数 `GetDeviceInfo(const char* pDeviceMac, SDeviceInfo& rDeviceInfo)` 和 `unsigned int GetNumberOfAttribute(const char* pDeviceMac)`,你可以使用 ctypes 库来进行 C 函数的调用。首先,需要导入 ctypes 模块,并加载包含这两个函数的共享库。
下面是一个示例代码,演示如何调用这两个函数:
```python
import ctypes
# 定义 SDeviceInfo 结构体
class SDeviceInfo(ctypes.Structure):
_fields_ = [("attribute1", ctypes.c_int),
("attribute2", ctypes.c_float),
# 添加其他属性字段的定义
("attributeN", ctypes.c_double)]
# 加载共享库
lib = ctypes.CDLL("your_shared_library.so") # 替换为你的共享库文件名
# 定义函数参数和返回类型
lib.GetDeviceInfo.argtypes = [ctypes.c_char_p, ctypes.POINTER(SDeviceInfo)] # 参数类型为 const char* 和 SDeviceInfo*
lib.GetDeviceInfo.restype = None
lib.GetNumberOfAttribute.argtypes = [ctypes.c_char_p] # 参数类型为 const char*
lib.GetNumberOfAttribute.restype = ctypes.c_uint # 返回类型为 unsigned int
# 创建 SDeviceInfo 实例
device_info = SDeviceInfo()
# 调用 GetDeviceInfo 函数
device_mac = b"device_mac" # 传递给函数的 const char* 参数,需要以字节串的形式提供
lib.GetDeviceInfo(device_mac, ctypes.byref(device_info))
# 打印设备信息的属性值
print(device_info.attribute1)
print(device_info.attribute2)
# 打印其他属性值
# 调用 GetNumberOfAttribute 函数
num_of_attributes = lib.GetNumberOfAttribute(device_mac)
# 打印属性数量
print(num_of_attributes)
```
请注意,在上面的示例中,你需要将 `"your_shared_library.so"` 替换为你实际使用的共享库的文件名。另外,根据实际情况,你可能需要调整函数的参数类型和返回类型,以及 SDeviceInfo 结构体的字段定义。
这是一个基本的示例,具体的实现可能因为你所使用的共享库和操作系统而有所不同。如果你能提供更多的上下文或代码片段,我可以帮助你更具体地解决这个问题。
相关推荐
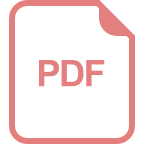
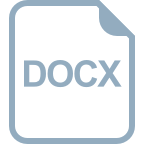
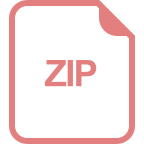












