Tesseract-ocr识别中文的python代码
时间: 2023-09-22 12:11:13 浏览: 140
使用Python进行Tesseract-ocr识别中文的代码如下:
```python
import pytesseract
from PIL import Image
# 安装好Tesseract-ocr,并设置环境变量
# 读入图片
img = Image.open('image.png')
# 转化为灰度图像
img = img.convert('L')
# 对图像进行二值化处理
threshold = 150
table = []
for i in range(256):
if i < threshold:
table.append(0)
else:
table.append(1)
img = img.point(table, '1')
# 调用Tesseract-ocr进行识别
result = pytesseract.image_to_string(img, lang='chi_sim')
print(result)
```
在代码中,首先需要导入pytesseract模块以及PIL库中的Image模块。然后读入需要识别的图片,将其转化为灰度图像,并进行二值化处理。最后调用Tesseract-ocr进行中文识别,将结果输出。
相关问题
Tesseract-OCR识别对图像进行处理代码
Tesseract OCR是一个开源的光学字符识别引擎,它能从图像文件或扫描文档中识别出文本内容。在Python中,我们可以利用`pytesseract`库来集成Tesseract OCR的功能。以下是基本的步骤:
```python
# 首先安装必要的库
!pip install pytesseract opencv-python Pillow
import cv2 # OpenCV用于读取和预处理图像
from PIL import Image # Python Imaging Library
import pytesseract
# 加载图像并转换为灰度
img = cv2.imread('image.jpg', cv2.IMREAD_GRAYSCALE)
# 使用PIL将OpenCV图像转换为Image对象
img_pil = Image.fromarray(img)
# 对图像进行增强,如二值化、去噪等,提高识别效果
config = '--psm 6' # 参数配置,PSM模式选择6表示页面分割
text = pytesseract.image_to_string(img_pil, config=config)
print(text) # 输出识别的文本
```
在这个代码中,我们首先加载图像,然后将其转换为灰度图以便于OCR识别。接着,我们使用`image_to_string()`函数进行识别,并可以自定义一些参数,比如`--psm`选项来调整识别模式。
tesseract-ocr python识别验证码
Tesseract OCR是一款开源的光学字符识别引擎,它常用于将图像中的文本转换成机器可读的文本格式。在Python中,你可以通过`pytesseract`库方便地集成Tesseract OCR。以下是基本步骤:
1. 安装所需库:首先需要安装`Pillow`和`tesseract`本身(如果你还没有安装的话)。在命令行中运行:
```
pip install Pillow pytesseract
```
对于Windows用户还需要下载Tesseract for Windows,并配置环境变量。
2. 导入库并导入Tesseract:在Python脚本中,引入`pytesseract`和`PIL`模块:
```python
import pytesseract
from PIL import Image
```
3. 加载图片:打开包含验证码的图片文件:
```python
image = Image.open('captcha_image.png')
```
4. 进行人脸检测和OCR识别:
```python
text = pytesseract.image_to_string(image, lang='chi_sim') # 'chi_sim'表示识别简体中文
```
5. 获取识别结果:
```python
print(text)
```
注意,验证码通常设计复杂,可能会有扭曲、噪声或者干扰线,识别率可能不高。提高识别成功率通常需要对图像预处理(如灰度化、二值化、降噪等),以及针对验证码特定的设计特征进行优化,比如使用专门的验证码识别模型。
阅读全文
相关推荐
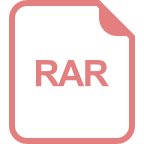
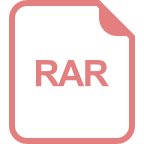
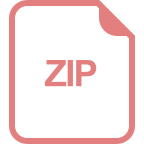
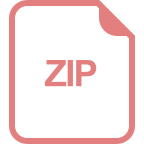
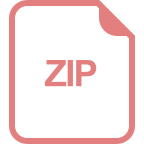
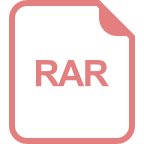
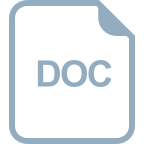





