torch.nn.conv2d 参数padding_mode
时间: 2023-09-13 19:05:33 浏览: 217
`padding_mode`是`torch.nn.conv2d`中的一个参数,用于设置在卷积操作中如何填充图像的边缘区域。
有两个选项可以选择:
- `'zeros'`:使用零来填充图像的边缘区域。
- `'reflect'`:使用图像的对称副本来填充边缘区域,这样可以避免出现人工填充时可能出现的边缘效应。
默认值为`'zeros'`。
相关问题
torch.nn.Conv2d的详细用法
`torch.nn.Conv2d`的详细用法如下:
```python
torch.nn.Conv2d(in_channels, out_channels, kernel_size, stride=1, padding=0, dilation=1, groups=1, bias=True, padding_mode='zeros')
```
其中各参数的含义如下:
- `in_channels`:输入通道数,即输入张量的深度。
- `out_channels`:输出通道数,即输出张量的深度,也就是卷积核的数量。
- `kernel_size`:卷积核大小,可以是一个整数,表示正方形卷积核的边长,也可以是一个元组,表示非正方形卷积核的宽和高。
- `stride`:卷积核的步长,默认为1。
- `padding`:输入张量四周的填充数,可以是一个整数,表示四周都填充相同的数量,也可以是一个元组,表示每个方向填充不同的数量。
- `dilation`:卷积核内部的扩张率,默认为1。
- `groups`:输入和输出通道之间的分组数,默认为1。
- `bias`:是否使用偏置项,默认为True。
- `padding_mode`:填充模式,默认为'zeros',表示用0进行填充,还可以使用'circular'表示用循环填充。
`torch.nn.Conv2d`的输入张量形状为`(batch_size, in_channels, height, width)`,输出张量形状为`(batch_size, out_channels, height_out, width_out)`,其中`height_out`和`width_out`分别是输出张量的高度和宽度,可以根据输入张量、卷积核大小、步长、填充等参数计算得到。
下面是一个示例:
```python
import torch
# 定义输入张量
x = torch.randn(1, 3, 32, 32)
# 定义卷积层
conv = torch.nn.Conv2d(3, 16, kernel_size=3, stride=1, padding=1)
# 进行卷积操作
out = conv(x)
# 输出张量形状
print(out.shape) # torch.Size([1, 16, 32, 32])
```
这个示例中,输入张量的形状为`(1, 3, 32, 32)`,表示批大小为1,通道数为3,高度和宽度均为32的输入张量。卷积核大小为3,分别在高度和宽度上进行1个像素的填充,步长为1,输出通道数为16,因此输出张量的形状为`(1, 16, 32, 32)`。
def fuse_conv_bn(self, conv, bn): std = (bn.running_var + bn.eps).sqrt() bias = bn.bias - bn.running_mean * bn.weight / std t = (bn.weight / std).reshape(-1, 1, 1, 1) weights = conv.weight * t bn = nn.Identity() conv = nn.Conv2d(in_channels=conv.in_channels, out_channels=conv.out_channels, kernel_size=conv.kernel_size, stride=conv.stride, padding=conv.padding, dilation=conv.dilation, groups=conv.groups, bias=True, padding_mode=conv.padding_mode) conv.weight = torch.nn.Parameter(weights) conv.bias = torch.nn.Parameter(bias) return conv 这个是上面提到的fuse_conv
`fuse_conv_bn` 是一个方法,它接受两个参数 `conv` 和 `bn`,分别代表卷积层和批归一化层。该方法的目的是将卷积层和批归一化层进行融合,并返回融合后的卷积层。
具体的实现步骤如下:
1. 计算批归一化层的标准差 `std`,其中 `bn.running_var` 是批归一化层的方差,`bn.eps` 是一个小的常数用于数值稳定性。
2. 计算偏置项 `bias`,通过批归一化层的均值 `bn.running_mean`、权重 `bn.weight` 和标准差 `std` 计算得到。
3. 根据标准差 `std` 对权重进行调整,将其乘以批归一化层的权重 `bn.weight`。
4. 创建一个新的卷积层对象 `conv`,与输入的卷积层 `conv` 具有相同的参数(通道数、核大小、步长等)。
5. 将调整后的权重赋值给新的卷积层对象的权重属性 `conv.weight`。
6. 将偏置项赋值给新的卷积层对象的偏置属性 `conv.bias`。
7. 返回融合后的卷积层对象 `conv`。
通过这个方法,可以实现将卷积层和批归一化层进行融合,减少网络的参数量和计算量。
相关推荐
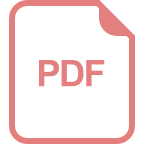
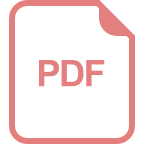
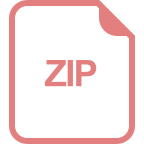












