用结构体编写一程序(应该有多个函数),允许从键盘输入任意多个英语单词(单词可以重复),中间用空格分开,输入0表示输入结束。该程序可以统计同一个英语单词被输入几次,最后对英文单词按字典顺序输出,后面跟上该单词被输入的次数。
时间: 2023-05-19 19:04:15 浏览: 76
好的,这是一个很好的编程问题。下面是一个示例程序,可以实现你所描述的功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_WORD_LEN 50
#define MAX_WORDS 1000
typedef struct {
char word[MAX_WORD_LEN];
int count;
} WordCount;
int compare_words(const void *a, const void *b) {
return strcmp(((WordCount *)a)->word, ((WordCount *)b)->word);
}
void count_words(WordCount *words, int *num_words) {
char word[MAX_WORD_LEN];
int i, j, found;
while (scanf("%s", word) == 1 && strcmp(word, "0") != 0) {
found = 0;
for (i = 0; i < *num_words; i++) {
if (strcmp(words[i].word, word) == 0) {
words[i].count++;
found = 1;
break;
}
}
if (!found) {
strcpy(words[*num_words].word, word);
words[*num_words].count = 1;
(*num_words)++;
}
}
qsort(words, *num_words, sizeof(WordCount), compare_words);
}
void print_words(WordCount *words, int num_words) {
int i;
for (i = 0; i < num_words; i++) {
printf("%s %d\n", words[i].word, words[i].count);
}
}
int main() {
WordCount words[MAX_WORDS];
int num_words = 0;
count_words(words, &num_words);
print_words(words, num_words);
return 0;
}
```
这个程序使用了一个结构体 `WordCount` 来保存每个单词及其出现次数。在 `count_words` 函数中,我们使用一个循环来读取输入的单词,然后在 `words` 数组中查找该单词是否已经存在。如果存在,就将其出现次数加一;否则,就将该单词添加到数组中,并将其出现次数设为一。最后,我们使用 `qsort` 函数对单词按字典顺序排序,并在 `print_words` 函数中输出每个单词及其出现次数。
希望这个程序能够满足你的需求!
阅读全文
相关推荐
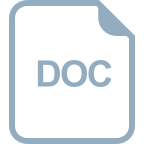
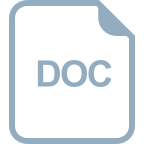
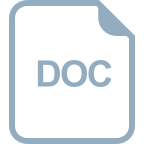







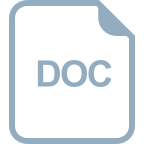
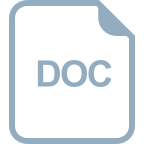
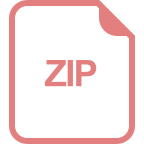
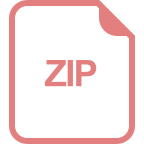
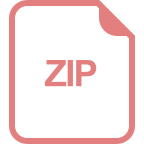