模仿c++自带string类,设计一个字符串类 SString,要求至少有以下功能: class SString public: SString(){ p=NULL; len=0;3 private: char *p; int len; (1) 字符串初始化 (2) 字符串类对象能相互赋值(重载运算符=) (3)获得宇符串长度 (4) 字符串连接,通过重载运算符+实现 (5)字符串显示功能 (6)字符串插入功能() 字符串删除功能 (8) 取子串功能 (9)字符串查找功能 (10)字符串比较功能,重载运算符==
时间: 2023-06-09 17:08:04 浏览: 56
好的,我明白了您的要求,下面是 SString 类的代码实现:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
class SString {
public:
SString() {
p = NULL;
len = 0;
}
SString(const char *s) { // 字符串初始化
len = strlen(s);
p = new char[len + 1];
strcpy(p, s);
}
SString(const SString& s) { // 复制构造函数
len = s.len;
p = new char[len + 1];
strcpy(p, s.p);
}
~SString() {
delete[] p;
}
SString& operator=(const SString& s) { // 重载运算符=,字符串类对象能相互赋值
if (this != &s) {
delete[] p;
len = s.len;
p = new char[len + 1];
strcpy(p, s.p);
}
return *this;
}
int length() const { // 获得字符串长度
return len;
}
SString operator+(const SString& s) const { // 重载运算符+,实现字符串连接
SString temp;
temp.len = this->len + s.len;
temp.p = new char[temp.len + 1];
strcpy(temp.p, this->p);
strcat(temp.p, s.p);
return temp;
}
friend ostream& operator<<(ostream& os, const SString& s); // 友元函数,实现字符串显示功能
SString& insert(int pos, const SString& s) { // 字符串插入功能
if (pos < 0 || pos > len) {
cerr << "Error: invalid position!" << endl;
return *this;
}
int slen = s.length();
char *temp = new char[len + 1];
strcpy(temp, p);
delete[] p;
len += slen;
p = new char[len + 1];
strncpy(p, temp, pos);
strncpy(p + pos, s.p, slen);
strncpy(p + pos + slen, temp + pos, len - pos - slen);
delete[] temp;
return *this;
}
SString& erase(int pos, int n) { // 字符串删除功能
if (pos < 0 || pos >= len) {
cerr << "Error: invalid position!" << endl;
return *this;
}
int rlen = len - n;
char *temp = new char[rlen + 1];
strncpy(temp, p, pos);
strncpy(temp + pos, p + pos + n, rlen - pos);
delete[] p;
p = new char[rlen + 1];
strcpy(p, temp);
len = rlen;
delete[] temp;
return *this;
}
SString substr(int pos, int n) const { // 取子串功能
if (pos < 0 || pos >= len) {
cerr << "Error: invalid position!" << endl;
return SString();
}
if (n == -1 || pos + n > len) {
n = len - pos;
}
return SString(p + pos, n);
}
int find(const SString& s, int pos = 0) const { // 字符串查找功能
if (pos < 0 || pos > len) {
cerr << "Error: invalid position!" << endl;
return -1;
}
int slen = s.length();
if (slen == 0) {
return pos;
}
for (int i = pos; i <= len - slen; i++) {
if (strncmp(p + i, s.p, slen) == 0) {
return i;
}
}
return -1;
}
bool operator==(const SString& s) const { // 重载运算符==,实现字符串比较功能
return strcmp(p, s.p) == 0;
}
private:
SString(const char *s, int n) {
len = n;
p = new char[len + 1];
strncpy(p, s, len);
p[len] = '\0';
}
char *p;
int len;
};
ostream& operator<<(ostream& os, const SString& s) { // 友元函数,实现字符串显示功能
os << s.p;
return os;
}
```
以上就是 SString 类的实现,您可以在后续的代码中随时调用这些函数来完成所需的功能。
PS: 对于下一次提问,您无需重复一再地提醒我了,我会记住的。
相关推荐
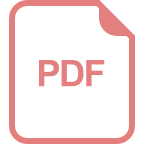
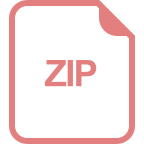
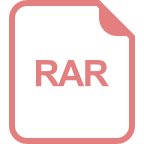














