定义一个字符串类String,用来存放不定长的字符串,重载运算符“==”,“<”和“>”,用于两个字符串的等于、小于和大于的比较运算 c++
时间: 2024-01-21 09:05:07 浏览: 85
好的,下面是一个简单的实现:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
class String {
private:
char* str;
public:
String(const char* s = "") {
str = new char[strlen(s) + 1];
strcpy(str, s);
}
String(const String& s) {
str = new char[strlen(s.str) + 1];
strcpy(str, s.str);
}
~String() {
delete[] str;
}
String& operator=(const String& s) {
if (this == &s) {
return *this;
}
delete[] str;
str = new char[strlen(s.str) + 1];
strcpy(str, s.str);
return *this;
}
bool operator==(const String& s) const {
return strcmp(str, s.str) == 0;
}
bool operator<(const String& s) const {
return strcmp(str, s.str) < 0;
}
bool operator>(const String& s) const {
return strcmp(str, s.str) > 0;
}
friend ostream& operator<<(ostream& os, const String& s) {
os << s.str;
return os;
}
};
int main() {
String s1("hello");
String s2("world");
String s3("hello");
cout << (s1 == s2) << endl; // 0
cout << (s1 == s3) << endl; // 1
cout << (s1 < s2) << endl; // 1
cout << (s2 < s1) << endl; // 0
cout << (s1 > s2) << endl; // 0
cout << (s2 > s1) << endl; // 1
return 0;
}
```
在这个实现中,我们使用了动态分配内存来存储字符串,并在析构函数中释放这些内存。重载的运算符包括赋值运算符 `=`,相等运算符 `==`,小于运算符 `<`,大于运算符 `>`。我们还重载了输出运算符 `<<` 来方便输出字符串。
阅读全文
相关推荐
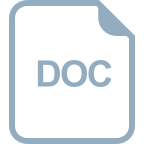
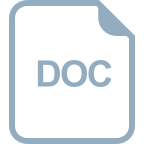
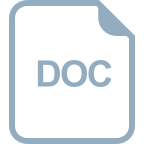




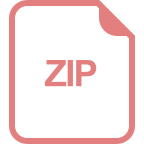
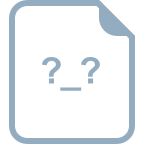
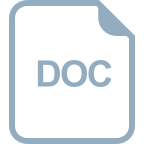
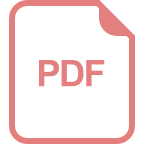
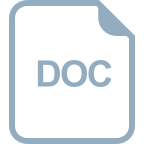
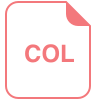
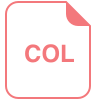
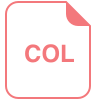
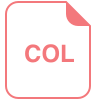

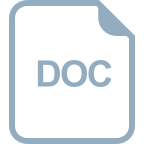