c语言取出字符串中的数字
时间: 2024-03-21 13:34:39 浏览: 75
在C语言中,可以使用sscanf函数来从字符串中提取数字。以下是一个示例代码:
```c
#include <stdio.h>
int main() {
char str[] = "123 45";
int x, y;
sscanf(str, "%d %d", &x, &y);
printf("x = %d\n", x);
printf("y = %d\n", y);
return 0;
}
```
上述代码中,我们定义了一个字符串`str`,其中包含两个数字。然后,我们使用sscanf函数从字符串中提取这两个数字,并将它们存储在变量`x`和`y`中。最后,我们使用printf函数将提取的数字打印出来。
运行上述代码,输出将是:
```
x = 123
y = 45
```
这样,我们就成功地从字符串中提取了数字。
相关问题
C语言如何从输入的字符串中提取数字
你可以使用 C语言 中的函数 `isdigit()` 来判断字符串中的每个字符是否是数字。具体步骤如下:
1. 定义一个字符数组来存储输入的字符串。
2. 使用 `scanf()` 函数从用户输入中读取字符串。
3. 遍历字符串中的每个字符,判断是否是数字。如果是数字,将其取出并存储到另一个数组中。
4. 最后输出存储数字的数组即可。
以下是代码示例:
```
#include <stdio.h>
#include <ctype.h>
int main() {
char str[50];
int num[50], i, j = 0;
printf("输入一个字符串:\n");
scanf("%s", str);
for (i = 0; str[i] != '\0'; i++) {
if (isdigit(str[i])) {
num[j++] = str[i] - '0';
}
}
printf("字符串中的数字为:\n");
for (i = 0; i < j; i++) {
printf("%d ", num[i]);
}
return 0;
}
```
怎么用c语言输出字符串的第一位的数字
假设你要输出字符串的第一位数字,可以使用以下代码:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "12345"; // 假设要输出的字符串为 "12345"
int num = str[0] - '0'; // 将字符串的第一位字符转换为数字(ASCII码减去0的ASCII码)
printf("第一位数字为:%d\n", num);
return 0;
}
```
运行结果为:
```
第一位数字为:1
```
解释一下代码:
首先定义一个字符串 `str`,并初始化为 "12345"。然后使用 `str[0]` 取出字符串的第一个字符,即 '1'。由于字符的 ASCII 码值可以通过减去 '0' 的 ASCII 码值得到对应的数字,因此使用 `str[0] - '0'` 将字符 '1' 转换为数字 1。最后使用 `printf` 输出结果。
相关推荐
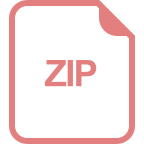
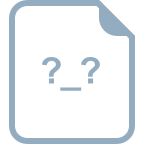












