pyhton插入sheet
时间: 2023-09-24 20:08:39 浏览: 41
要使用Python插入Excel中的工作表,需要使用`openpyxl`库。下面是一个简单的例子:
```python
import openpyxl
# 打开工作簿
wb = openpyxl.load_workbook('example.xlsx')
# 创建新的工作表
new_sheet = wb.create_sheet('New Sheet')
# 将数据写入新工作表
new_sheet['A1'] = 'Hello'
new_sheet['B1'] = 'World'
# 保存工作簿
wb.save('example.xlsx')
```
在这个例子中,我们首先使用`openpyxl.load_workbook()`打开现有的Excel工作簿。然后使用`wb.create_sheet()`创建一个新的工作表,并将其分配给`new_sheet`变量。最后,我们将数据写入新工作表中,并使用`wb.save()`保存工作簿。
需要注意的是,`openpyxl`只支持`.xlsx`格式的Excel文件。如果您正在使用`.xls`文件,则需要使用不同的库,例如`xlwt`。
相关问题
python插入excel
在Python中插入Excel数据可以使用`openpyxl`库。首先,你需要安装`openpyxl`库,可以使用以下命令进行安装:
```
pip install openpyxl
```
然后,你可以使用以下代码示例来插入数据到Excel文件中:
```python
from openpyxl import Workbook
# 创建一个新的工作簿
workbook = Workbook()
# 选择活动的工作表
sheet = workbook.active
# 插入数据到单元格
sheet['A1'] = 'Hello'
sheet['B1'] = 'World'
# 保存工作簿
workbook.save('example.xlsx')
```
上面的代码将在当前目录下创建一个名为`example.xlsx`的Excel文件,并在第一行的两个单元格中插入数据。
你可以根据需要修改代码以插入更多的数据或者指定不同的单元格位置。
Python插入excel
### 使用Python库将数据插入Excel文件
#### 利用 `openpyxl` 库操作 Excel 文件
为了利用 `openpyxl` 插入数据至 Excel 文件,先要安装此库。可以通过 pip 安装工具来完成这一过程。
```bash
pip install openpyxl
```
下面是一个简单的例子展示如何创建一个新的工作簿并写入一些数据:
```python
from openpyxl import Workbook
wb = Workbook() # 创建新的工作簿对象
ws = wb.active # 获取活动的工作表
ws.title = "Sample Sheet"
data_to_insert = [
["Name", "Age", "City"],
["Alice", 30, "New York"],
["Bob", 24, "Los Angeles"]
]
for row in data_to_insert:
ws.append(row)
wb.save("sample.xlsx") # 将更改保存到新文件中
```
这段代码会生成一个名为 `sample.xlsx` 的文件,在其中含有两列三行的数据[^1]。
#### 借助 `pandas` 和 `openpyxl` 处理更复杂的情况
对于结构化数据而言,比如来自数据库查询的结果或者是 CSV 文件中的表格形式的数据集,可以借助于 `pandas` 来简化处理流程。Pandas 提供了一个非常方便的方法叫做 `to_excel()` 可以直接把 DataFrame 对象导出成 Excel 表格。
首先同样需要确保已经安装了必要的包:
```bash
pip install pandas openpyxl
```
接着看一段具体的实例代码如下所示:
```python
import pandas as pd
df = pd.DataFrame({
'Names': ['Charlie', 'David'],
'Ages': [28, 29],
'Cities': ['Chicago', 'Houston']
})
with pd.ExcelWriter('output.xlsx', engine='openpyxl') as writer:
df.to_excel(writer, sheet_name='People Info', index=False)
```
这里通过 `pd.ExcelWriter` 方法指定了使用的引擎为 `openpyxl` 并设置了目标文件名以及工作表名称;而 `index=False` 参数则表示不需要写出索引列[^2]。
阅读全文
相关推荐



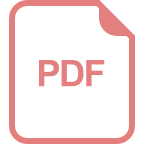












