明文加密移3位c语言
时间: 2024-10-28 18:12:07 浏览: 20
明文加密移位(也称为凯撒密码)是一种简单的替换式密码,它通过将字母按照固定的位数向右移动来加密文本。在C语言中实现这种操作,你可以创建一个函数,接受明文、密钥和字符集作为输入。以下是一个基本的示例:
```c
#include <stdio.h>
#include <string.h>
char shift_encrypt(char c, int key) {
// A-Z范围,+1是因为'A'对应ASCII值0
if ('A' <= c && c <= 'Z') {
return ((c - 'A' + key) % 26) + 'A';
}
// a-z范围
else if ('a' <= c && c <= 'z') {
return ((c - 'a' + key) % 26) + 'a';
}
// 如果不是字母,则保持原样
else {
return c;
}
}
void encrypt_string(const char *plaintext, int key, char encrypted[]) {
for (int i = 0; plaintext[i] != '\0'; ++i) {
encrypted[i] = shift_encrypt(plaintext[i], key);
}
}
int main() {
const char *plain_text = "Hello, World!";
int key = 3;
char encrypted_text[50];
encrypt_string(plain_text, key, encrypted_text);
printf("Plain Text: %s\n", plain_text);
printf("Encrypted Text: %s\n", encrypted_text);
return 0;
}
```
这个程序会将给定的明文字符串`plain_text`中的每个字符按指定的键(如3位)向右移动并存储到`encrypted_text`中。
阅读全文
相关推荐
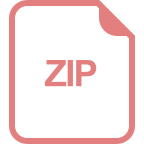
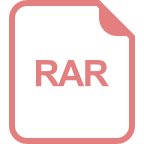


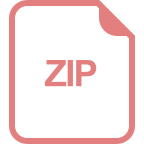
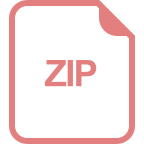
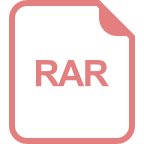
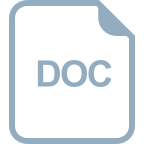
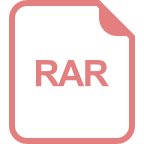
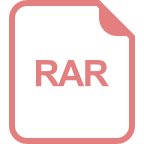
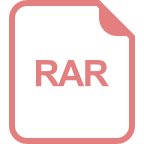
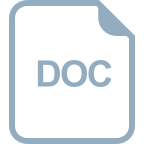
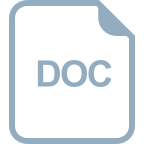
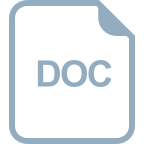
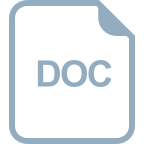
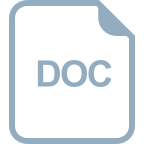

