凯撒密码加密解密替换算法C语言
时间: 2023-10-19 20:07:17 浏览: 154
凯撒密码是一种简单的加密算法,它的原理是将明文中的每个字母按照一定的偏移量进行替换,从而得到密文。具体来说,假设偏移量为k,明文中的每个字母都向后移动k个位置,如果超出了字母表的范围,则从头开始循环。例如,当k=3时,明文中的字母A会被替换成D,B会被替换成E,以此类推。
下面是一个简单的C语言实现:
```c
#include <stdio.h>
#include <string.h>
void encrypt(char *plaintext, int k) {
int len = strlen(plaintext);
for (int i = 0; i < len; i++) {
if (plaintext[i] >= 'A' && plaintext[i] <= 'Z') {
plaintext[i] = (plaintext[i] - 'A' + k) % 26 + 'A'; } else if (plaintext[i] >= 'a' && plaintext[i] <= 'z') {
plaintext[i] = (plaintext[i] - 'a' + k) % 26 + 'a';
}
}
}
void decrypt(char *ciphertext, int k) {
int len = strlen(ciphertext);
for (int i = 0; i < len; i++) {
if (ciphertext[i] >= 'A' && ciphertext[i] <= 'Z') {
ciphertext[i] = (ciphertext[i] - 'A' - k + 26) % 26 + 'A';
} else if (ciphertext[i] >= 'a' && ciphertext[i] <= 'z') {
ciphertext[i] = (ciphertext[i] - 'a' - k + 26) % 26 + 'a';
}
}
}
int main() {
char plaintext[100], ciphertext[100];
int k;
printf("请输入明文:");
scanf("%s", plaintext);
printf("请输入偏移量:");
scanf("%d", &k);
encrypt(plaintext, k);
printf("加密后的密文为:%s\n", plaintext);
decrypt(plaintext, k);
printf("解密后的明文为:%s\n", plaintext);
return 0;
}
```
这个程序中,encrypt函数用于加密明文,decrypt函数用于解密密文。它们都接受两个参数:一个是要加密/解密的字符串,另一个是偏移量k。在加密过程中,我们首先计算出每个字母在字母表中的位置,然后将其向后移动k个位置,最后再将其转换成对应的字符。在解密过程中,我们需要将每个字母向前移动k个位置,这可以通过将加密过程中的加法变成减法来实现。
相关推荐
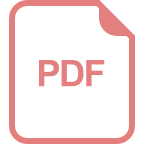














