java凯撒密码加密解密算法
时间: 2023-12-22 08:29:42 浏览: 103
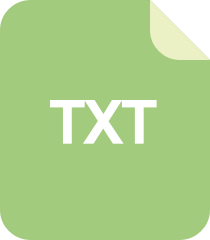
凯撒密码的加密解密算法
以下是Java中实现凯撒密码加密解密算法的示例代码:
```java
public class CaesarCipher {
// 加密函数
public static String encrypt(String plaintext, int shift) {
StringBuilder ciphertext = new StringBuilder();
for (int i = 0; i < plaintext.length(); i++) {
char ch = plaintext.charAt(i);
if (Character.isLetter(ch)) {
if (Character.isUpperCase(ch)) {
char encryptedChar = (char) ((ch - 'A' + shift) % 26 + 'A');
ciphertext.append(encryptedChar);
} else {
char encryptedChar = (char) ((ch - 'a' + shift) % 26 + 'a');
ciphertext.append(encryptedChar);
}
} else {
ciphertext.append(ch);
}
}
return ciphertext.toString();
}
// 解密函数
public static String decrypt(String ciphertext, int shift) {
StringBuilder plaintext = new StringBuilder();
for (int i = 0; i < ciphertext.length(); i++) {
char ch = ciphertext.charAt(i);
if (Character.isLetter(ch)) {
if (Character.isUpperCase(ch)) {
char decryptedChar = (char) ((ch - 'A' - shift + 26) % 26 + 'A');
plaintext.append(decryptedChar);
} else {
char decryptedChar = (char) ((ch - 'a' - shift + 26) % 26 + 'a');
plaintext.append(decryptedChar);
}
} else {
plaintext.append(ch);
}
}
return plaintext.toString();
}
public static void main(String[] args) {
String plaintext = "Hello, World!";
int shift = 3;
String ciphertext = encrypt(plaintext, shift);
System.out.println("Encrypted text: " + ciphertext);
String decryptedText = decrypt(ciphertext, shift);
System.out.println("Decrypted text: " + decryptedText);
}
}
```
阅读全文
相关推荐
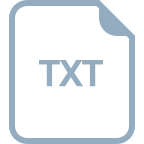
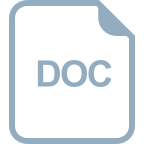
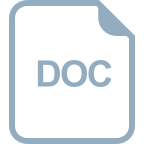





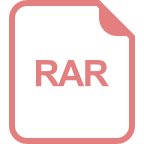
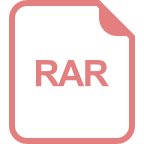
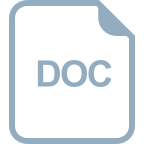



