凯撒密码加密解密算法
时间: 2023-10-19 16:06:49 浏览: 240
凯撒密码是一种替换加密的技术,明文中的所有字母都在字母表上向后(或向前)按照一个固定数目进行偏移后被替换成密文。例如,当偏移量是3的时候,所有的字母A将被替换成D,B变成E,以此类推。在代码实现中,可以利用输入的字母进行加减,如果超过了字母表的范围,则循环回到字母表的开头或结尾。
凯撒密码的加密解密算法可以通过以下步骤实现:
1. 获取用户输入的加密或解密操作以及相应的文本。
2. 获取用户输入的偏移量,确保偏移量在合理范围内(0-25)。
3. 根据用户选择的操作进行相应的处理:
- 加密操作:遍历文本中的每个字符,如果是字母,根据偏移量进行向后移位,并考虑循环回到字母表的开头或结尾。
- 解密操作:遍历文本中的每个字符,如果是字母,根据偏移量进行向前移位,并考虑循环回到字母表的开头或结尾。
4. 输出加密或解密后的结果。
以下是一个用C语言实现凯撒密码加密解密算法的示例代码:
```
#include <stdio.h>
#include <string.h>
void caesarEncrypt(char text[], int shift) {
int length = strlen(text);
for (int i = 0; i < length; i++) {
if ((text[i] >= 'a' && text[i] <= 'z') || (text[i] >= 'A' && text[i] <= 'Z')) {
if (text[i] >= 'a' && text[i] <= 'z') {
text[i] = 'a' + (text[i] - 'a' + shift) % 26; }
if (text[i] >= 'A' && text[i] <= 'Z') {
text[i] = 'A' + (text[i] - 'A' + shift) % 26;
}
}
}
}
void caesarDecrypt(char text[], int shift) {
caesarEncrypt(text, 26 - shift);
}
int main() {
char text[1000];
int shift, option;
printf("请输入加密(1)或解密(2):");
scanf("%d", &option);
getchar(); // 读取换行符
printf("请输入文本:");
fgets(text, sizeof(text), stdin);
text[strcspn(text, "\n")] = '\0'; // 去除换行符
printf("请输入偏移量:");
scanf("%d", &shift);
if (option == 1) {
caesarEncrypt(text, shift);
printf("加密后的文本:%s\n", text);
} else if (option == 2) {
caesarDecrypt(text, shift);
printf("解密后的文本:%s\n", text);
} else {
printf("无效的操作!\n");
}
return 0;
}
```
阅读全文
相关推荐
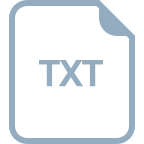
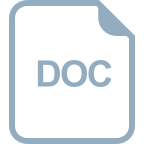





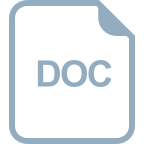

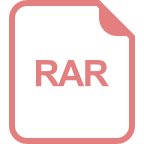
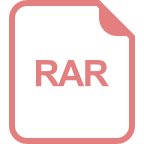
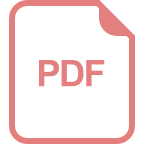


