请你根据我给出的代码片段,帮我转换成pytorch模型
时间: 2024-12-14 15:15:50 浏览: 4
好的,以下是将提供的Keras代码片段转换为PyTorch版本的基本步骤:
```python
import torch.nn as nn
import torch
# 注意力块函数的转换
class AttentionBlock(nn.Module):
def __init__(self, feature_cnt, dim):
super(AttentionBlock, self).__init__()
self.feature_cnt = feature_cnt
self.dim = dim
self.fc = nn.Linear(self.feature_cnt * dim, feature_cnt * dim)
self.softmax = nn.Softmax(dim=1)
self.reshape = nn.Sequential(nn.Flatten(), nn.Linear(dim, (feature_cnt, dim)))
self.reduce_dim = nn.Linear(dim, 1, bias=False)
def forward(self, inputs):
attention_weights = self.fc(inputs).reshape(-1, self.feature_cnt, self.dim)
attention_weights = self.softmax(attention_weights)
attention_weights = attention_weights.unsqueeze(2)
attention_out = torch.bmm(inputs, attention_weights)
return attention_out
# Pixel Branch的转换
class PixelBranch(nn.Module):
def __init__(self):
super(PixelBranch, self).__init__()
self.conv_layers = nn.ModuleList([
nn.Conv1d(8, 16, kernel_size=11, stride=1, padding=(11 - 1) // 2),
AttentionBlock(170, 64),
nn.Conv1d(8+170, 16, kernel_size=3, stride=1, padding=1),
nn.ReLU(),
nn.MaxPool1d(kernel_size=2, stride=2),
nn.Conv1d(16, 16, kernel_size=3, stride=1, padding=1),
nn.ReLU(),
nn.MaxPool1d(kernel_size=2, stride=2),
nn.Conv1d(16, 16, kernel_size=3, stride=1, padding=1),
nn.ReLU(),
nn.MaxPool1d(kernel_size=2, stride=2),
nn.Flatten()
])
def forward(self, input_tensor):
for layer in self.conv_layers:
input_tensor = layer(input_tensor)
return input_tensor
# 创建PyTorch模型实例
model = PixelBranch()
```
这段代码定义了一个名为`PixelBranch`的PyTorch模块,包含了与Keras代码类似的结构,包括1D卷积、注意力块、非线性激活、池化和展平操作。请注意,在实际使用中,您可能需要根据具体需求进行进一步的修改和调整。
阅读全文
相关推荐
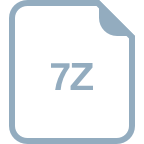
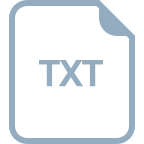
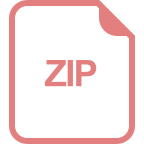
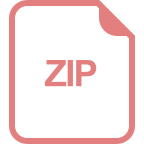
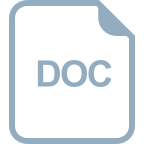
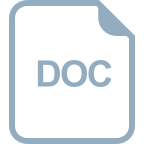
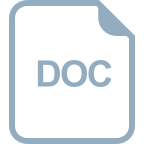
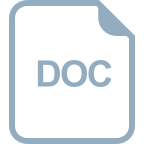
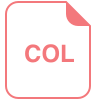
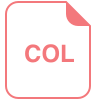
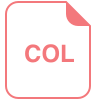
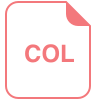






