Java判断目录权限_Java验证一个文件或目录是否有读写权限
时间: 2024-01-16 09:02:47 浏览: 84
可以使用Java的File类的方法来判断一个文件或目录是否有读写权限。具体实现如下:
判断文件或目录是否可读:
```java
File file = new File("path/to/file_or_directory");
if (file.canRead()) {
System.out.println(file.getAbsolutePath() + " is readable.");
} else {
System.out.println(file.getAbsolutePath() + " is not readable.");
}
```
判断文件或目录是否可写:
```java
File file = new File("path/to/file_or_directory");
if (file.canWrite()) {
System.out.println(file.getAbsolutePath() + " is writable.");
} else {
System.out.println(file.getAbsolutePath() + " is not writable.");
}
```
判断文件或目录是否可执行:
```java
File file = new File("path/to/file_or_directory");
if (file.canExecute()) {
System.out.println(file.getAbsolutePath() + " is executable.");
} else {
System.out.println(file.getAbsolutePath() + " is not executable.");
}
```
需要注意的是,以上方法只能判断文件或目录的权限是否可读、可写或可执行,无法判断具体的读、写或执行权限。如果需要判断具体的权限,可以使用Java的nio包中的Files类的方法。
阅读全文
相关推荐
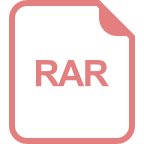
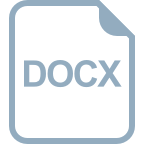
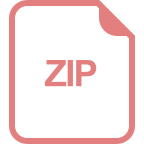
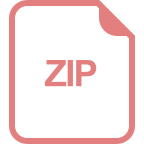
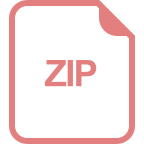
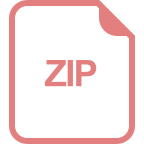
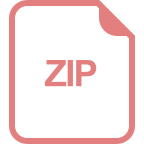
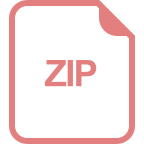
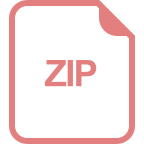
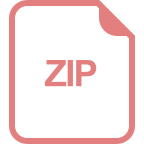