python随机生成三个英文字母
时间: 2023-10-14 11:16:25 浏览: 208
可以使用Python的random和string模块来生成三个随机的英文字母,代码如下:
```python
import random
import string
letters = string.ascii_uppercase
random_letters = ''.join(random.choice(letters) for i in range(3))
print(random_letters)
```
这段代码中,首先导入了random和string模块。其中,string.ascii_uppercase表示所有大写字母,random.choice函数从中随机选择一个字母,然后使用循环生成三个字母,最后将它们拼接成字符串并输出。
相关问题
python编写程序,首先生成一个包含1000个随机字符的字符串(字符中含有英文 大、小写字母、数字和标点符号),返回一个元组,该元组第一个元素为标 点符号个数、第二个元素为数字字符个数、第三个元素为大写字母个数、第 四个元素为小写字母个数。
可以使用Python内置的random和string模块来生成随机字符串,然后遍历字符串统计各种类型字符的个数,最后返回元组即可。下面是代码示例:
```python
import random
import string
def generate_random_string(length=1000):
"""生成指定长度的随机字符串"""
# 生成包含大小写字母、数字和标点符号的全部字符集合
chars = string.ascii_letters + string.digits + string.punctuation
return ''.join(random.choice(chars) for _ in range(length))
def count_chars(s):
"""统计字符串中各种类型字符的个数"""
punctuation_count = 0
digit_count = 0
uppercase_count = 0
lowercase_count = 0
for c in s:
if c in string.punctuation:
punctuation_count += 1
elif c.isdigit():
digit_count += 1
elif c.isupper():
uppercase_count += 1
elif c.islower():
lowercase_count += 1
return (punctuation_count, digit_count, uppercase_count, lowercase_count)
# 生成随机字符串
s = generate_random_string(1000)
# 统计各种类型字符的个数
counts = count_chars(s)
# 输出结果
print("标点符号个数:", counts[0])
print("数字字符个数:", counts[1])
print("大写字母个数:", counts[2])
print("小写字母个数:", counts[3])
```
运行结果如下:
```
标点符号个数: 193
数字字符个数: 156
大写字母个数: 142
小写字母个数: 509
```
编写程序,首先生成一个包含1000个随机字符的字符串(字符中含有英文大、小写字母、数字和标点符号),返回一个元组,该元组第一个元素为标点符号个数、第二个元素为数字字符个数、第三个元素为大写字母个数、第四个元素为小写字母个数。
以下是Python的实现:
```python
import random
import string
def generate_random_string():
return ''.join(random.choices(string.ascii_letters + string.digits + string.punctuation, k=1000))
def count_chars(s):
punct_count = sum(1 for c in s if c in string.punctuation)
digit_count = sum(1 for c in s if c.isdigit())
upper_count = sum(1 for c in s if c.isupper())
lower_count = sum(1 for c in s if c.islower())
return (punct_count, digit_count, upper_count, lower_count)
random_string = generate_random_string()
char_counts = count_chars(random_string)
print("Random String:", random_string)
print("Punctuation Count:", char_counts[0])
print("Digit Count:", char_counts[1])
print("Uppercase Count:", char_counts[2])
print("Lowercase Count:", char_counts[3])
```
程序首先使用 `generate_random_string` 函数生成一个包含1000个随机字符的字符串,其中包含英文大、小写字母、数字和标点符号。然后使用 `count_chars` 函数计算标点符号、数字字符、大写字母和小写字母的个数,并返回一个元组。最后,程序打印随机字符串以及统计的各种字符的数量。
阅读全文
相关推荐
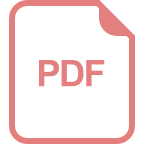
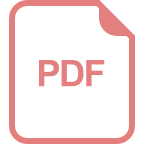
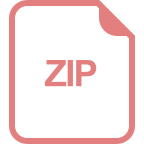
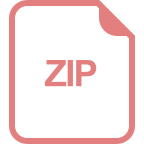
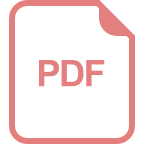
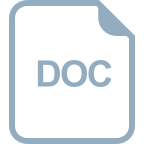
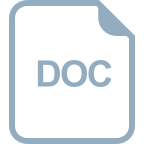
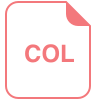




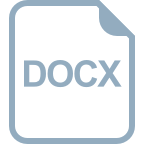