基于高斯过程的贝叶斯优化python代码
时间: 2023-11-13 15:09:41 浏览: 47
以下是一个基于高斯过程的贝叶斯优化的 Python 代码示例,使用了 SciPy 和 Scikit-learn 库:
```python
import numpy as np
from scipy.optimize import minimize
from sklearn.gaussian_process import GaussianProcessRegressor
from sklearn.gaussian_process.kernels import Matern
# 定义目标函数
def target_function(x):
return np.sin(5 * x) * (1 - np.tanh(x ** 2)) + np.random.randn() * 0.1
# 定义贝叶斯优化函数
def bayesian_optimization(func, bounds, n_iters):
x = np.random.uniform(bounds[:, 0], bounds[:, 1], size=(n_iters, bounds.shape[0]))
y = np.array([func(xx) for xx in x])
kernel = Matern(length_scale=1.0, nu=2.5)
gp = GaussianProcessRegressor(kernel=kernel, alpha=1e-5, n_restarts_optimizer=5, random_state=0)
for _ in range(n_iters):
gp.fit(x, y)
mu, sigma = gp.predict(x, return_std=True)
next_x = np.argmax(mu)
next_y = func(x[next_x])
x = np.vstack([x, next_x])
y = np.append(y, next_y)
return x[np.argmin(y)]
# 定义参数边界
bounds = np.array([[-5, 5]])
# 运行贝叶斯优化
result = bayesian_optimization(target_function, bounds, n_iters=20)
print("最小值所在位置:", result)
print("最小值:", target_function(result))
```
在这个例子中,我们使用了 Matern 核函数,该函数可以适应不同长度尺度的特征,并且可以控制平滑度。我们通过随机生成一些初始点并计算它们的函数值来初始化高斯过程回归器。在每次迭代中,我们使用高斯过程回归器来预测最可能的下一个点,并将其添加到之前的点中,以便我们可以计算新的函数值。通过多次迭代,我们不断优化目标函数并找到最小值。
相关推荐
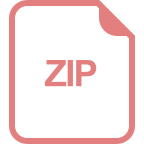
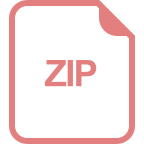
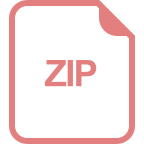














