python实现图像识别阴影部分的代码编写
时间: 2023-07-23 15:05:06 浏览: 157
实现图像识别阴影部分的方法有很多种,下面我提供一种基于OpenCV库的方法。具体步骤如下:
1. 读入图像并转换为灰度图像。
```python
import cv2
img = cv2.imread('image.jpg')
gray_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
```
2. 对灰度图像进行二值化处理,将图像转换为黑白图像。
```python
threshold_img = cv2.threshold(gray_img, 0, 255, cv2.THRESH_BINARY_INV+cv2.THRESH_OTSU)[1]
```
3. 对二值化后的图像进行形态学操作,填充图像中的小孔和缺陷。
```python
kernel = cv2.getStructuringElement(cv2.MORPH_RECT, (3,3))
morph_img = cv2.morphologyEx(threshold_img, cv2.MORPH_CLOSE, kernel)
```
4. 找到图像中所有连通区域。
```python
cnts, hierarchy = cv2.findContours(morph_img, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
```
5. 遍历所有连通区域,如果该区域的面积小于一定阈值,则将其填充为白色(即去除阴影)。
```python
threshold_area = 100 # 面积阈值
for cnt in cnts:
area = cv2.contourArea(cnt)
if area < threshold_area:
cv2.drawContours(morph_img, [cnt], 0, 255, -1)
```
最终得到的 `morph_img` 就是去除了阴影的图像。完整代码如下:
```python
import cv2
img = cv2.imread('image.jpg')
gray_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
threshold_img = cv2.threshold(gray_img, 0, 255, cv2.THRESH_BINARY_INV+cv2.THRESH_OTSU)[1]
kernel = cv2.getStructuringElement(cv2.MORPH_RECT, (3,3))
morph_img = cv2.morphologyEx(threshold_img, cv2.MORPH_CLOSE, kernel)
cnts, hierarchy = cv2.findContours(morph_img, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
threshold_area = 100 # 面积阈值
for cnt in cnts:
area = cv2.contourArea(cnt)
if area < threshold_area:
cv2.drawContours(morph_img, [cnt], 0, 255, -1)
cv2.imshow('image', morph_img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
阅读全文
相关推荐
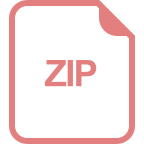
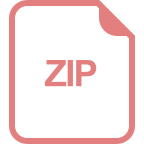
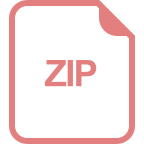
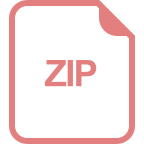
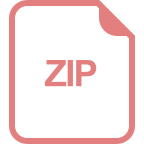
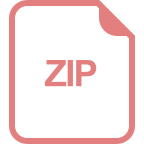
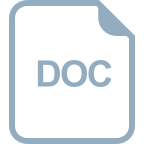
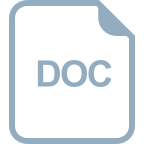
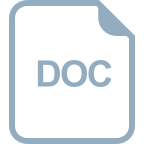
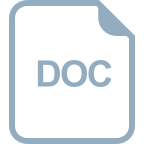
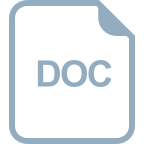
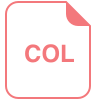
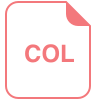
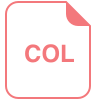
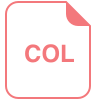
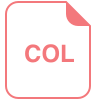
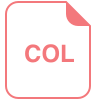
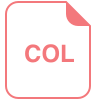