roberts算子Prewitt算子Sobel算子Laplacian算子的比较
时间: 2023-09-05 09:11:00 浏览: 130
Robert算子、Prewitt算子、Sobel算子、Laplacian算子都是常见的边缘检测算子,它们的原理和使用方式略有不同,下面是它们的比较:
1. Robert算子:计算速度快,但对噪声敏感,容易产生误检测。
2. Prewitt算子:计算速度较快,对噪声的抑制效果较好,但边缘检测的结果不如Sobel算子和Laplacian算子。
3. Sobel算子:与Prewitt算子类似,但对噪声的抑制效果更好,且可以通过改变卷积核的大小来调整边缘检测的灵敏度。
4. Laplacian算子:可以检测出更多的边缘信息,但容易受到噪声的影响,且会产生较多的假阳性结果。
在实际应用中,选择哪种算子需要根据具体情况而定。如果需要快速计算边缘信息,可以选择Robert算子或Sobel算子;如果需要更准确的边缘信息,可以选择Laplacian算子;如果需要在较强噪声的情况下进行边缘检测,可以选择Prewitt算子或Sobel算子。
相关问题
使用MATLAB实现Roberts算子、Prewitt算子、Sobel算子、Laplacian算子、Canny算子的对比程序
以下是使用MATLAB实现Roberts算子、Prewitt算子、Sobel算子、Laplacian算子、Canny算子的对比程序:
```matlab
% 读入图像
img = imread('lena.png');
% 转为灰度图像
img_gray = rgb2gray(img);
%% Roberts算子
% 定义Roberts算子的模板
roberts_mat = [-1 0; 0 1];
% 应用Roberts算子
img_roberts = imfilter(double(img_gray), roberts_mat);
% 显示结果
figure; imshow(uint8(img_roberts)); title('Roberts算子');
%% Prewitt算子
% 定义Prewitt算子的模板
prewitt_mat = [-1 -1 -1; 0 0 0; 1 1 1];
% 应用Prewitt算子
img_prewitt = imfilter(double(img_gray), prewitt_mat);
% 显示结果
figure; imshow(uint8(img_prewitt)); title('Prewitt算子');
%% Sobel算子
% 定义Sobel算子的模板
sobel_mat = [-1 -2 -1; 0 0 0; 1 2 1];
% 应用Sobel算子
img_sobel = imfilter(double(img_gray), sobel_mat);
% 显示结果
figure; imshow(uint8(img_sobel)); title('Sobel算子');
%% Laplacian算子
% 定义Laplacian算子的模板
laplacian_mat = [0 -1 0; -1 4 -1; 0 -1 0];
% 应用Laplacian算子
img_laplacian = imfilter(double(img_gray), laplacian_mat);
% 显示结果
figure; imshow(uint8(img_laplacian)); title('Laplacian算子');
%% Canny算子
% 应用Canny算子
img_canny = edge(img_gray, 'canny');
% 显示结果
figure; imshow(img_canny); title('Canny算子');
```
运行上述代码,可以得到Roberts、Prewitt、Sobel、Laplacian和Canny算子处理后的图像。你可以将代码中的`lena.png`替换为你自己的图像,并调整算子的参数进行实验。
使用MATLAB实现Roberts算子、Prewitt算子、Sobel算子、Laplacian算子、Canny算子的程序
以下是使用MATLAB实现Roberts算子、Prewitt算子、Sobel算子、Laplacian算子、Canny算子的程序。
Roberts算子:
```matlab
% 读取图像
img = imread('image.jpg');
% 将图像转化为灰度图像
gray_img = rgb2gray(img);
% Roberts算子
roberts_x = [1 0; 0 -1];
roberts_y = [0 1; -1 0];
% 计算x方向和y方向的梯度
roberts_grad_x = conv2(double(gray_img), roberts_x, 'same');
roberts_grad_y = conv2(double(gray_img), roberts_y, 'same');
% 计算梯度的幅值
roberts_grad = sqrt(roberts_grad_x.^2 + roberts_grad_y.^2);
% 显示结果
imshow(uint8(roberts_grad));
```
Prewitt算子:
```matlab
% 读取图像
img = imread('image.jpg');
% 将图像转化为灰度图像
gray_img = rgb2gray(img);
% Prewitt算子
prewitt_x = [-1 0 1; -1 0 1; -1 0 1];
prewitt_y = [-1 -1 -1; 0 0 0; 1 1 1];
% 计算x方向和y方向的梯度
prewitt_grad_x = conv2(double(gray_img), prewitt_x, 'same');
prewitt_grad_y = conv2(double(gray_img), prewitt_y, 'same');
% 计算梯度的幅值
prewitt_grad = sqrt(prewitt_grad_x.^2 + prewitt_grad_y.^2);
% 显示结果
imshow(uint8(prewitt_grad));
```
Sobel算子:
```matlab
% 读取图像
img = imread('image.jpg');
% 将图像转化为灰度图像
gray_img = rgb2gray(img);
% Sobel算子
sobel_x = [-1 0 1; -2 0 2; -1 0 1];
sobel_y = [-1 -2 -1; 0 0 0; 1 2 1];
% 计算x方向和y方向的梯度
sobel_grad_x = conv2(double(gray_img), sobel_x, 'same');
sobel_grad_y = conv2(double(gray_img), sobel_y, 'same');
% 计算梯度的幅值
sobel_grad = sqrt(sobel_grad_x.^2 + sobel_grad_y.^2);
% 显示结果
imshow(uint8(sobel_grad));
```
Laplacian算子:
```matlab
% 读取图像
img = imread('image.jpg');
% 将图像转化为灰度图像
gray_img = rgb2gray(img);
% Laplacian算子
laplacian = [-1 -1 -1; -1 8 -1; -1 -1 -1];
% 计算梯度
laplacian_grad = conv2(double(gray_img), laplacian, 'same');
% 显示结果
imshow(uint8(laplacian_grad));
```
Canny算子:
```matlab
% 读取图像
img = imread('image.jpg');
% 将图像转化为灰度图像
gray_img = rgb2gray(img);
% Canny算子
canny_grad = edge(gray_img, 'canny');
% 显示结果
imshow(canny_grad);
```
相关推荐
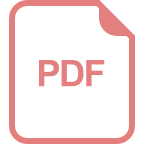
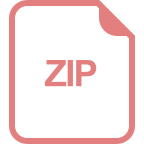












