用python读取图像barbara.nmp,采用Laplacian算子,RObert算子,Prewitt算子和sobel算子进行图像锐化边缘处理,并比较说明锐化效果
时间: 2024-09-24 11:06:00 浏览: 95
在Python中,我们可以使用OpenCV库来读取图像并应用各种边缘检测算子,如Laplacian、Roberts、Prewitt和Sobel。以下是简单的步骤:
首先,你需要安装`opencv-python`库,如果尚未安装,可以使用pip安装:
```bash
pip install opencv-python
```
然后,你可以按照以下步骤操作:
1. **读取图像**:
```python
import cv2
image = cv2.imread('barbara.png', cv2.IMREAD_GRAYSCALE) # 读取灰度图像
if image is None:
print("无法打开图片")
else:
height, width = image.shape
```
2. **应用算子**:
- **Laplacian算子**:
```python
laplacian = cv2.Laplacian(image, cv2.CV_64F)
```
- **Roberts算子**:
```python
Roberts_x = np.array([[1, 0], [0, -1]])
Roberts_y = np.array([[-1, 0], [0, 1]])
Roberts = cv2.filter2D(image, -1, Roberts_x) + cv2.filter2D(image, -1, Roberts_y)
```
- **Prewitt算子** 和 **Sobel算子** 的计算类似,但OpenCV内置函数更方便:
```python
prewitt = cv2.Prewitt(image, ddepth=cv2.CV_64F)
sobelx = cv2.Sobel(image, cv2.CV_64F, dx=1, dy=0, ksize=3)
sobely = cv2.Sobel(image, cv2.CV_64F, dx=0, dy=1, ksize=3)
```
3. **对比显示**:
```python
images_to_compare = [laplacian, Roberts, prewitt, sobelx, sobely]
for i, img in enumerate(images_to_compare):
cv2.imshow(f"Image {i+1}: Edge Detection", img)
cv2.waitKey(0) # 等待用户按键
cv2.destroyAllWindows()
```
**锐化效果比较**:
- Laplacian算子对细节变化敏感,但可能会引入噪声。
- Roberts算子简单易懂,适用于小范围边缘,有时结果不如其他算子清晰。
- Prewitt和Sobel算子提供了一定的方向性,Sobel还允许自定义方向,结果通常比Roberts更精确。
注意:这些操作的结果会依赖于原始图像内容,对于不同的图像,锐化效果可能会有所不同。
阅读全文
相关推荐






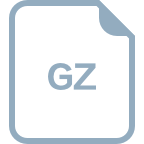




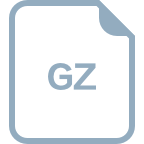


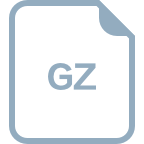


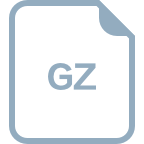