读取图像Barbara.bmp,基于OpenCV设计滤波器模板为椭圆(a/b=2)的理想低通滤波与高通滤波,显示原始图像,滤波后的频谱图和高低通滤波处理后的图像。python代码
时间: 2024-10-06 21:06:50 浏览: 60
首先,我们需要导入OpenCV和一些额外的库来帮助我们完成这个任务。以下是一个基本的Python代码示例,它展示了如何读取图像、应用理想低通和高通滤波,以及显示结果。
```python
import cv2
import numpy as np
from matplotlib import pyplot as plt
# 读取图像
image_path = 'Barbara.bmp'
img = cv2.imread(image_path, cv2.IMREAD_GRAYSCALE)
# 确保图像为单通道
if len(img.shape) == 3:
img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 检查图像是否加载成功
if img is None:
print("Image not loaded.")
else:
# 设定滤波器参数
a = 2
b = a / 2
center_x, center_y = img.shape[1] // 2, img.shape[0] // 2
radius = int(min(a, b) * img.shape[0]) // 2 # 计算滤波器半径
# 创建理想低通滤波模板
low_pass_kernel = np.zeros((radius*2+1, radius*2+1), dtype=np.float32)
low_pass_kernel[radius, radius] = 1
# 创建理想高通滤波模板
high_pass_kernel = np.copy(low_pass_kernel)
high_pass_kernel[radius, radius] = -1
# 应用滤波
filtered_img_lowpass = cv2.filter2D(img, -1, low_pass_kernel)
filtered_img_highpass = cv2.filter2D(img, -1, high_pass_kernel)
# 频谱图展示
f = np.fft.fft2(img)
fshift = np.fft.fftshift(f)
magnitude_spectrum = 20 * np.log(np.abs(fshift))
plt.figure(figsize=(15, 6))
plt.subplot(1, 4, 1), plt.imshow(img, cmap='gray'), plt.title('Original Image')
plt.subplot(1, 4, 2), plt.imshow(magnitude_spectrum, cmap='gray'), plt.title('Magnitude Spectrum')
plt.subplot(1, 4, 3), plt.imshow(filtered_img_lowpass, cmap='gray'), plt.title('Low Pass Filtered Image')
plt.subplot(1, 4, 4), plt.imshow(filtered_img_highpass, cmap='gray'), plt.title('High Pass Filtered Image')
plt.show()
阅读全文
相关推荐
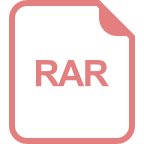
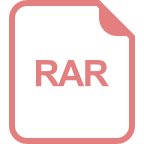
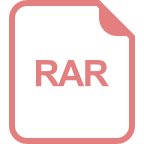

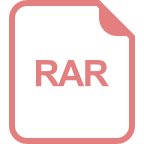
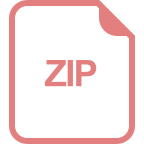
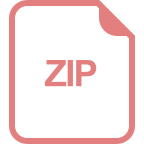
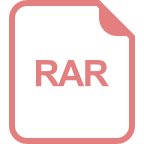
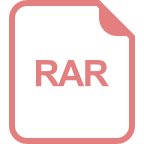
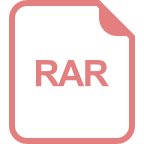
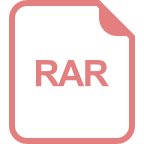
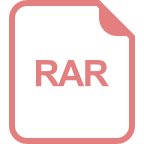
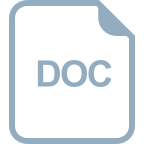
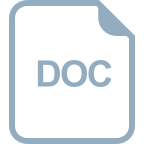


