运用 MyBatis,mysql做一个student表的增加并测试
时间: 2024-03-27 14:35:13 浏览: 15
好的,下面是使用 MyBatis 和 MySQL 创建 student 表并进行增加操作的步骤:
1. 创建数据库和表
首先需要创建一个数据库,例如名为 `mybatis_test`,然后创建一个名为 `student` 的表,表结构如下:
```sql
CREATE TABLE `student` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(20) DEFAULT NULL,
`age` int(11) DEFAULT NULL,
`gender` varchar(10) DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
```
2. 配置 MyBatis
在项目中引入 MyBatis 相关依赖,并且在 `resources` 目录下创建 `mybatis-config.xml` 文件,配置 MyBatis 的基本信息和数据源信息,例如:
```xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<environments default="development">
<environment id="development">
<transactionManager type="JDBC"/>
<dataSource type="POOLED">
<property name="driver" value="com.mysql.jdbc.Driver"/>
<property name="url" value="jdbc:mysql://localhost:3306/mybatis_test"/>
<property name="username" value="root"/>
<property name="password" value="root"/>
</dataSource>
</environment>
</environments>
<mappers>
<mapper resource="mapper/StudentMapper.xml"/>
</mappers>
</configuration>
```
其中 `StudentMapper.xml` 文件是后面要编写的映射文件,这里先引入。
3. 编写实体类
创建一个名为 `Student` 的 Java 实体类,包含 `id`、`name`、`age` 和 `gender` 四个属性,并且提供相应的 getter 和 setter 方法。
4. 编写 Mapper 接口和映射文件
创建一个名为 `StudentMapper` 的 Mapper 接口,定义增加学生信息的方法:
```java
public interface StudentMapper {
void addStudent(Student student);
}
```
然后,在 `resources` 目录下创建 `mapper` 文件夹,并且在其中创建 `StudentMapper.xml` 文件,编写 Mapper 接口的映射信息:
```xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.example.mapper.StudentMapper">
<insert id="addStudent" parameterType="com.example.entity.Student" useGeneratedKeys="true" keyProperty="id">
insert into student(name, age, gender)
values(#{name}, #{age}, #{gender})
</insert>
</mapper>
```
其中 `namespace` 属性指定了 Mapper 接口的全限定名,`id` 属性指定了方法名,`parameterType` 属性指定了方法参数类型。`useGeneratedKeys` 属性表示使用数据库的自增主键,`keyProperty` 属性指定了实体类中与主键对应的属性名。
5. 编写测试代码
创建一个名为 `StudentMapperTest` 的测试类,编写测试代码:
```java
@RunWith(SpringRunner.class)
@SpringBootTest
public class StudentMapperTest {
@Autowired
private StudentMapper studentMapper;
@Test
public void testAddStudent() {
Student student = new Student();
student.setName("Tom");
student.setAge(18);
student.setGender("Male");
studentMapper.addStudent(student);
Assert.assertNotNull(student.getId());
System.out.println("新增学生信息成功,id=" + student.getId());
}
}
```
在测试方法中创建一个学生对象,并且调用 Mapper 接口的 `addStudent` 方法进行添加操作。添加成功后,断言主键不为空,并且输出新增学生信息的主键。
6. 运行测试
运行测试方法,如果一切正常,会输出新增学生信息的主键。此时可以去数据库中查询,可以看到已经成功添加了一条学生记录。
这就是使用 MyBatis 和 MySQL 创建 student 表并进行增加操作的全部步骤。
相关推荐
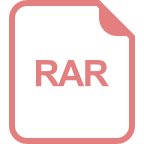
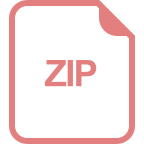














