公司现有以下设备:普通电视机、DVD、 收藏 带DVD 的电视机,将这三个设备提前存进文件中。并且条件是 带DVD 的电视机的售价为普通电视机和 DVD 单价之和的 80%。 要求对所有的库存设备都能按照品 名进行显示、修改、查找、增加、删除、保存设备信息、的功能,先写主函数 然后在编写每个函数 函数简单 尽量有注释 用c语言编写 不使用指针和链表
时间: 2024-02-04 08:03:06 浏览: 135
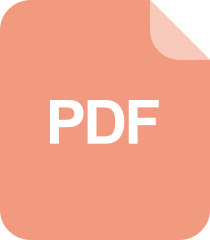
数字电视机顶盒中Java虚拟机的研究
好的,以下是一个简单的控制台程序,实现了对设备信息的基本操作。请注意,为了简化程序,我将设备信息存储在一个全局数组中,而没有使用文件进行读写。在实际应用中,应该将设备信息存储在文件中,并从文件中读取和保存信息。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_DEVICES 100
// 设备结构体
typedef struct {
char name[20]; // 品名
int price; // 价格
int has_dvd; // 是否带DVD,1表示带DVD,0表示不带
} Device;
// 设备列表
Device devices[MAX_DEVICES];
int num_devices = 0; // 当前设备数量
// 显示所有设备信息
void list_devices() {
printf("当前设备数量:%d\n", num_devices);
printf("品名\t价格\t是否带DVD\n");
for (int i = 0; i < num_devices; i++) {
printf("%s\t%d\t%s\n", devices[i].name, devices[i].price, devices[i].has_dvd ? "是" : "否");
}
}
// 查找设备
int find_device(const char* name) {
for (int i = 0; i < num_devices; i++) {
if (strcmp(devices[i].name, name) == 0) {
return i;
}
}
return -1;
}
// 添加设备
void add_device() {
if (num_devices >= MAX_DEVICES) {
printf("设备数量已达到上限,无法添加新设备!\n");
return;
}
Device device;
printf("请输入设备品名:");
scanf("%s", device.name);
printf("请输入设备价格:");
scanf("%d", &device.price);
printf("该设备是否带DVD?(1表示是,0表示否):");
scanf("%d", &device.has_dvd);
// 计算带DVD电视机的价格
if (strcmp(device.name, "带DVD电视机") == 0) {
int tv_price, dvd_price;
for (;;) {
printf("请输入普通电视机的价格:");
scanf("%d", &tv_price);
printf("请输入DVD的价格:");
scanf("%d", &dvd_price);
if (tv_price + dvd_price > 0) {
break;
}
printf("价格必须大于0,请重新输入!\n");
}
device.price = (int)(tv_price + dvd_price) * 0.8;
printf("带DVD电视机的价格已自动计算为:%d\n", device.price);
}
devices[num_devices++] = device;
printf("设备已添加成功!\n");
}
// 修改设备信息
void modify_device() {
char name[20];
printf("请输入需要修改的设备品名:");
scanf("%s", name);
int index = find_device(name);
if (index == -1) {
printf("设备不存在!\n");
return;
}
Device* device = &devices[index];
printf("当前设备信息:\n");
printf("品名:%s\n", device->name);
printf("价格:%d\n", device->price);
printf("是否带DVD:%s\n", device->has_dvd ? "是" : "否");
// 修改价格
printf("请输入新的价格(如不需要修改,请输入0):");
int new_price;
scanf("%d", &new_price);
if (new_price > 0) {
device->price = new_price;
}
// 修改是否带DVD
printf("该设备是否带DVD?(1表示是,0表示否,如不需要修改,请输入-1):");
int has_dvd;
scanf("%d", &has_dvd);
if (has_dvd == 0 || has_dvd == 1) {
device->has_dvd = has_dvd;
}
printf("设备信息已修改成功!\n");
}
// 删除设备
void delete_device() {
char name[20];
printf("请输入需要删除的设备品名:");
scanf("%s", name);
int index = find_device(name);
if (index == -1) {
printf("设备不存在!\n");
return;
}
// 将最后一个设备覆盖要删除的设备
devices[index] = devices[num_devices - 1];
num_devices--;
printf("设备已删除!\n");
}
// 保存设备信息到文件
void save_devices() {
FILE* fp = fopen("devices.txt", "w");
if (fp == NULL) {
printf("无法打开文件!\n");
return;
}
fprintf(fp, "%d\n", num_devices);
for (int i = 0; i < num_devices; i++) {
fprintf(fp, "%s %d %d\n", devices[i].name, devices[i].price, devices[i].has_dvd);
}
fclose(fp);
printf("设备信息已保存到文件!\n");
}
// 从文件中加载设备信息
void load_devices() {
FILE* fp = fopen("devices.txt", "r");
if (fp == NULL) {
printf("无法打开文件!\n");
return;
}
fscanf(fp, "%d", &num_devices);
for (int i = 0; i < num_devices; i++) {
fscanf(fp, "%s %d %d", devices[i].name, &devices[i].price, &devices[i].has_dvd);
}
fclose(fp);
printf("设备信息已从文件中加载!\n");
}
// 主函数
int main() {
load_devices();
printf("欢迎使用设备管理系统!\n");
for (;;) {
printf("请输入操作代码(1-显示设备列表,2-添加设备,3-修改设备信息,4-删除设备,5-保存设备信息到文件,0-退出):");
int code;
scanf("%d", &code);
switch (code) {
case 1:
list_devices();
break;
case 2:
add_device();
break;
case 3:
modify_device();
break;
case 4:
delete_device();
break;
case 5:
save_devices();
break;
case 0:
printf("感谢使用设备管理系统,再见!\n");
return 0;
default:
printf("无效的操作代码,请重新输入!\n");
break;
}
}
}
```
阅读全文
相关推荐
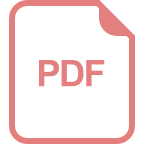
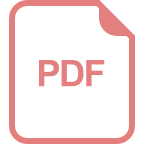















