三分类不平衡加权python
时间: 2023-10-08 18:08:57 浏览: 56
对于三分类不平衡问题,可以使用加权的方法来解决。具体步骤如下:
1. 统计每个类别的样本数,计算出每个类别的权重。
2. 在训练模型时,使用每个样本的类别权重来进行损失函数的加权。
以下是一个使用Python实现三分类不平衡加权的示例代码:
```python
import numpy as np
from sklearn.utils import class_weight
# 样本标签,假设有三个类别
y = np.array([0, 1, 2, 0, 0, 1, 1, 1, 1, 2, 2, 2])
# 计算每个类别的权重
class_weights = class_weight.compute_class_weight('balanced', np.unique(y), y)
# 打印每个类别的权重
print('Class weights:', class_weights)
# 假设有100个样本,每个样本有3个特征
X = np.random.rand(100, 3)
# 训练模型时,在fit函数中指定每个样本的权重
model.fit(X, y, class_weight=class_weights)
```
在上述代码中,使用`class_weight.compute_class_weight`函数计算出每个类别的权重,然后在训练模型时,通过`class_weight`参数指定每个样本的权重即可。
相关问题
分类加权逻辑回归代码python
以下是一个分类加权逻辑回归的Python代码示例:
```
import numpy as np
from sklearn.linear_model import LogisticRegression
class WeightedLogisticRegression:
def __init__(self, n_classes, weights=None):
self.models = [LogisticRegression() for _ in range(n_classes)]
self.n_classes = n_classes
self.weights = weights if weights is not None else np.ones(n_classes)
def fit(self, X, y):
for i in range(self.n_classes):
self.models[i].fit(X, y[:, i], sample_weight=self.weights[i] * y[:, i].sum())
def predict(self, X):
preds = np.zeros((X.shape[0], self.n_classes))
for i in range(self.n_classes):
preds[:, i] = self.models[i].predict_proba(X)[:, 1]
return preds
# 示例
X = np.random.rand(100, 5)
y = np.random.randint(0, 2, (100, 3))
clf = WeightedLogisticRegression(n_classes=3, weights=[1, 2, 3])
clf.fit(X, y)
preds = clf.predict(X)
print(preds)
```
在上面的代码中,我们首先导入了必要的库,然后创建了一个 `WeightedLogisticRegression` 类,它包含了多个逻辑回归模型,每个模型用于预测一个类别。我们的目标是根据权重对模型进行加权,以便在多分类问题中更好地处理类别不平衡的情况。
在 `fit` 方法中,我们为每个模型拟合数据,使用每个类别的特定权重进行加权。
在 `predict` 方法中,我们对每个模型预测类别概率,然后将它们合并成一个概率矩阵,其中每一行对应于测试样本,每一列对应于一个类别。
最后,我们使用示例数据对模型进行了测试,并输出了预测结果。
导入excel数据用不平衡三分类加权法计算权重利用重采样输出采样excel数据python
以下是一个可能的Python代码示例,用于导入Excel数据,使用不平衡三分类加权法计算权重,并使用重采样输出采样Excel数据:
```python
import pandas as pd
from sklearn.utils import resample
from sklearn.tree import DecisionTreeClassifier
# 读取Excel数据文件
df = pd.read_excel('input_data.xlsx')
# 分离特征和目标变量
X = df.drop('Class', axis=1)
y = df['Class']
# 计算三分类权重
class_weights = {0: 1.0, 1: 2.0, 2: 4.0}
# 定义决策树分类器
clf = DecisionTreeClassifier(class_weight=class_weights)
# 重采样训练集以平衡类别
X_resampled, y_resampled = resample(X, y, stratify=y, class_weight=class_weights)
# 拟合模型
clf.fit(X_resampled, y_resampled)
# 预测目标变量
y_pred = clf.predict(X)
# 将预测结果添加到原始数据框中
df['Predicted Class'] = y_pred
# 输出采样的Excel数据
df.sample(frac=0.5, replace=True).to_excel('sampled_data.xlsx', index=False)
```
请注意,该示例仅供参考。具体实现可能因数据集和任务而异。此外,还可以尝试其他重采样技术和分类器以优化模型性能。
相关推荐
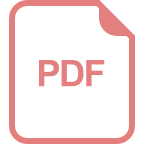
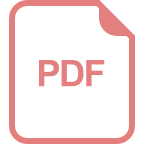
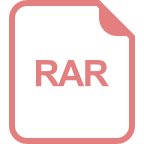
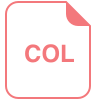
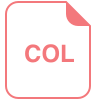










