let s= this.myArray[0]; 获取不到数值
时间: 2024-04-01 22:34:58 浏览: 18
如果您使用 `let s = this.myArray[0]` 获取不到数值,可能是因为 `this.myArray` 数组中没有任何元素,或者 `this.myArray` 并不存在。
请确保 `this.myArray` 数组已经被正确地初始化,并且至少包含一个元素。您可以在调试过程中使用 `console.log(this.myArray)` 打印出 `this.myArray` 数组的值,来检查其是否被正确地初始化。
如果 `this.myArray` 数组确实存在并且包含元素,但是您仍然无法获取到其中的数值,那么可能是因为元素的类型不正确。请确保您获取到的元素类型是您预期的类型,并且可以正确地进行操作。
另外,如果您使用的是异步代码,例如 AJAX 请求等,在获取 `this.myArray` 数组中的元素时,可能需要等待异步操作完成后才能获取到正确的结果。在这种情况下,您可以使用 Promise 或者 async/await 等方式来处理异步操作,确保在异步操作完成后才进行获取元素的操作。
相关问题
解释一下let newArray = myArray.slice(0, 1).concat(myArray.slice(2));
这段代码的作用是从数组 `myArray` 中删除索引为 1 的元素,并将结果存储在新数组 `newArray` 中。
让我们逐步解释这段代码:
1. `myArray.slice(0, 1)`:这一部分使用 `slice()` 方法从 `myArray` 中提取索引为 0 的元素,也就是第一个元素。这将返回一个只包含一个元素的新数组。
2. `.concat()`:这是数组的方法,用于连接两个或多个数组。在这里,它将连接前面提取的第一个元素数组和下一步要提取的剩余元素数组。
3. `myArray.slice(2)`:这一部分使用 `slice()` 方法从 `myArray` 中提取从索引为 2 开始到数组末尾的所有元素。这将返回一个新数组,包含从索引 2 开始的所有元素。
所以,通过将第一步提取的第一个元素数组和第三步提取的剩余元素数组拼接在一起,我们得到了一个不包含索引为 1 的元素的新数组 `newArray`。
例如,如果 `myArray` 是 `[1, 2, 3, 4, 5]`,那么执行以上代码后,`newArray` 将变为 `[1, 3, 4, 5]`。
希望这个解释对你有帮助!如有其他问题,请随时提问。
微信小程序中使用let myArray = [0x41, 0x02, 0x17, 0x05, 0x13, 0x09, 0x06, 0x05, 0x35, 0x7E, 0x00];let buf = Buffer.from(myArray);that.data.client.publish('Liang_tx', buf);,报错Buffer is not defined
在微信小程序中,Node.js的Buffer模块并不支持,需要使用小程序原生的API进行转换。可以通过以下代码实现:
```js
let myArray = [0x41, 0x02, 0x17, 0x05, 0x13, 0x09, 0x06, 0x05, 0x35, 0x7E, 0x00];
let typedArray = new Uint8Array(myArray);
let buf = typedArray.buffer;
let dataView = new DataView(buf);
let hexString = '';
for (let i = 0; i < typedArray.byteLength; i++) {
let str = dataView.getUint8(i).toString(16);
str = str.length === 2 ? str : '0' + str;
hexString += str;
}
that.data.client.publish('Liang_tx', hexString);
```
其中,`typedArray`是将数组转换成TypedArray类型,`buf`是将TypedArray类型转换成ArrayBuffer类型,`dataView`是通过ArrayBuffer类型创建DataView类型,`hexString`是将DataView中的数据转换成16进制字符串。最后将`hexString`作为参数传入`publish`方法即可。
相关推荐
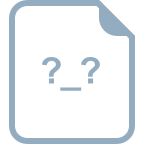












