#pragma once #include<iostream> using namespace std; template<class T> class MyArray { public: // 有参构造 MyAddress(int capacity) { this->m_Capacity = capacity; this->m_Size = 0; this->pAddress = new T[this->m_Capacity]; } // 析构函数 ~MyArray() { if (this->pAddress != NULL) { delete[]this->pAddress; this->MyAddress = NULL; } } // 拷贝构造 MyArray(const MyArray& arr) { this->m_Capacity = arr.m_Capacity; this->m_Size = arr.m_Size; /*this->pAddress = arr.pAddress;*/// 浅拷贝 this->pAddress = new T[arr.m_Capacity];// 深拷贝 // 将arr中的数据都拷贝过来 for (int i = 0; i < this->m_Size; i++) { this->pAddress[i] = arr.pAddress[i]; } } // operator= 防止浅拷贝问题 MyArray& operator=(const MyArray& arr) { // 判断原来堆区是否有数据,如果有先释放 if (this->pAddress != NULL) { delete[]this->pAddress; this->pAddress = NULL; this->m_Capacity = 0; this->m_Size = 0; } // 深拷贝 this->m_Capacity = arr.m_Capacity; this->m_Size = arr.m_Size; this->pAddress=new T[arr.m_Capacity] for (int i = 0; i < this->m_Size; i++) { this->pAddress[i] = arr.pAddress[i]; } return *this; } private: T* pAddress;// 指针指向堆区开辟的真实数组 int m_Capacity;// 数组容量 int m_Size;//数组大小 };上述代码有什么问题?
时间: 2024-04-16 19:27:25 浏览: 94
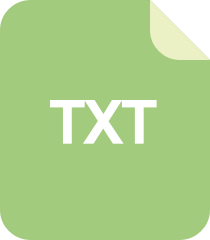
使用include <iostream>时一定要加using namespace std
在上述代码中,`MyArray` 类中的构造函数被命名为 `MyAddress`,而不是 `MyArray`,这是一个拼写错误。
此外,在析构函数中,将 `this->MyAddress` 设置为 `NULL` 是错误的,应该是将 `this->pAddress` 设置为 `NULL` 来释放堆内存。
在赋值操作符重载函数中,没有为 `pAddress` 分配新的内存空间之前就进行了赋值操作,这会导致潜在的内存泄漏。应该先为 `pAddress` 分配新的内存空间,然后再进行赋值操作。
修正后的代码如下:
```cpp
#pragma once
#include<iostream>
using namespace std;
template<class T>
class MyArray {
public:
// 有参构造
MyArray(int capacity) {
this->m_Capacity = capacity;
this->m_Size = 0;
this->pAddress = new T[this->m_Capacity];
}
// 析构函数
~MyArray() {
if (this->pAddress != NULL) {
delete[] this->pAddress;
this->pAddress = NULL;
}
}
// 拷贝构造
MyArray(const MyArray& arr) {
this->m_Capacity = arr.m_Capacity;
this->m_Size = arr.m_Size;
this->pAddress = new T[arr.m_Capacity];
// 将arr中的数据都拷贝过来
for (int i = 0; i < this->m_Size; i++) {
this->pAddress[i] = arr.pAddress[i];
}
}
// operator= 防止浅拷贝问题
MyArray& operator=(const MyArray& arr) {
// 判断原来堆区是否有数据,如果有先释放
if (this->pAddress != NULL) {
delete[] this->pAddress;
this->pAddress = NULL;
this->m_Capacity = 0;
this->m_Size = 0;
}
// 深拷贝
this->m_Capacity = arr.m_Capacity;
this->m_Size = arr.m_Size;
this->pAddress = new T[arr.m_Capacity];
for (int i = 0; i < this->m_Size; i++) {
this->pAddress[i] = arr.pAddress[i];
}
return *this;
}
private:
T* pAddress; // 指针指向堆区开辟的真实数组
int m_Capacity; // 数组容量
int m_Size; // 数组大小
};
```
修正后的代码中,`MyArray` 类的构造函数被改回为 `MyArray`,修复了拼写错误。同时,在析构函数和赋值操作符重载函数中,修正了错误的内存释放和深拷贝实现。
阅读全文
相关推荐
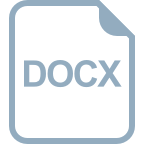
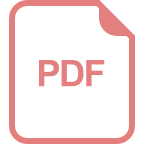











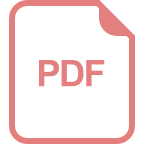
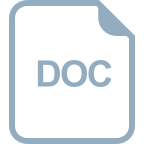
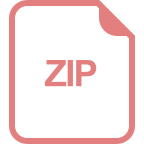
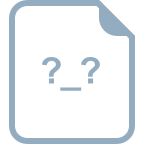