用c++写
时间: 2023-07-01 07:26:55 浏览: 71
好的,下面给出完整的 C++ 代码实现:
```c++
#include <iostream>
template <typename T>
class Node {
public:
T data;
Node<T>* next;
Node(T data) {
this->data = data;
next = nullptr;
}
};
template <typename T>
class LinkedList {
private:
Node<T>* head;
Node<T>* tail;
public:
LinkedList() {
head = nullptr;
tail = nullptr;
}
LinkedList(const LinkedList<T>& other) {
head = nullptr;
tail = nullptr;
Node<T>* current = other.head;
while (current != nullptr) {
append(current->data);
current = current->next;
}
}
void append(T data) {
Node<T>* node = new Node<T>(data);
if (head == nullptr) {
head = node;
tail = node;
}
else {
tail->next = node;
tail = node;
}
}
void print() {
Node<T>* current = head;
while (current != nullptr) {
std::cout << current->data << " ";
current = current->next;
}
std::cout << std::endl;
}
};
int main() {
LinkedList<int> list1;
list1.append(1);
list1.append(2);
list1.append(3);
list1.print();
LinkedList<int> list2(list1);
list2.print();
return 0;
}
```
在这个例子中,我们先定义了一个`Node`类,表示单链表的节点,包含数据`data`和指向下一个节点的指针`next`。然后定义了`LinkedList`类,表示单链表,包含头指针`head`和尾指针`tail`,并且定义了构造函数、拷贝构造函数、添加节点的方法和打印链表的方法。
在`main`函数中,我们先创建了一个新的链表`list1`,并向其中添加了三个节点。然后我们使用拷贝构造函数创建了另一个链表`list2`,并打印出两个链表的内容。可以发现,`list2`与`list1`的内容完全相同,说明拷贝构造函数成功地实现了链表的拷贝。
阅读全文
相关推荐
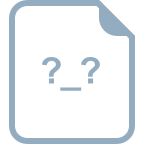
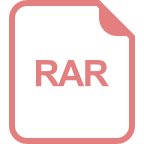
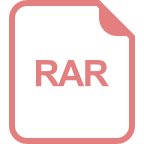
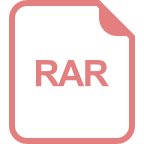
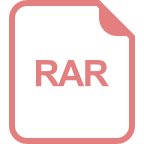
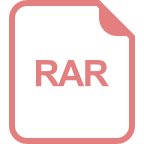
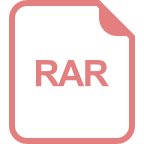