用C语言实现递归下降语法分析器
时间: 2023-11-18 16:28:32 浏览: 167
好的,以下是一个简单的递归下降语法分析器的C语言实现,用于解析上面提到的文法:
```c
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
char lookahead; // 当前字符
char input[100]; // 输入字符串
int pos = 0; // 当前字符位置
void error() {
printf("Syntax Error\n");
exit(1);
}
void match(char c) {
if (lookahead == c) {
lookahead = input[++pos];
} else {
error();
}
}
void E();
void E_();
void T() {
if (isdigit(lookahead)) {
printf("%c", lookahead);
match(lookahead);
} else if (lookahead == '(') {
match('(');
E();
match(')');
} else {
error();
}
}
void T_() {
if (lookahead == '*') {
match('*');
T();
T_();
} else if (lookahead == '/') {
match('/');
T();
T_();
}
}
void E() {
T();
E_();
}
void E_() {
if (lookahead == '+') {
match('+');
T();
E_();
} else if (lookahead == '-') {
match('-');
T();
E_();
}
}
int main() {
printf("Enter an arithmetic expression: ");
fgets(input, 100, stdin);
lookahead = input[pos];
E();
if (lookahead != '\n') {
error();
} else {
printf("\nSyntax is correct\n");
}
return 0;
}
```
该分析器使用递归下降的方式实现了对输入字符串的语法分析,如果输入的字符串符合文法规则,则输出其解析结果,并提示语法正确;否则输出“Syntax Error”提示语法错误。
阅读全文
相关推荐




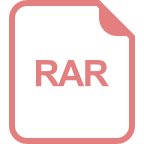






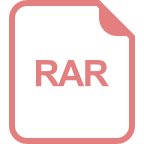
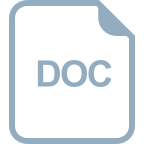



