java获得一年后的今天
时间: 2023-11-29 08:11:40 浏览: 41
您好!感谢您的提问。下面是 Java 获取一年后的今天的代码:
```java
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
public class Main {
public static void main(String[] args) {
// 获取当前日期
LocalDate currentDate = LocalDate.now();
// 计算一年后的日期
LocalDate nextYearDate = currentDate.plusYears(1);
// 格式化日期输出
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd");
System.out.println("一年后的今天是:" + nextYearDate.format(formatter));
}
}
```
代码中使用了 Java 8 中的 LocalDate 类来获取当前日期和计算一年后的日期,并使用 DateTimeFormatter 类来格式化输出。如果您使用的是 Java 8 之前的版本,可以使用 Calendar 类或者 Date 类来实现同样的功能。
希望能够帮到您!如果您有其他问题,可以继续提出。
相关问题
java获取一年后的今天
可以使用Java 8中的LocalDate类来获取一年后的今天。以下是示例代码:
```
LocalDate today = LocalDate.now();
LocalDate nextYearToday = today.plusYears(1);
```
其中,`LocalDate.now()`获取当前日期,`plusYears(1)`方法将当前日期加上1年,得到一年后的今天。
java 获取当前时间上一年
要获取当前时间的上一年,可以使用Java中的Calendar类或LocalDate类来实现。
使用Calendar类的示例代码如下:
```java
Calendar ca = Calendar.getInstance();
ca.setTime(new Date());
ca.add(Calendar.YEAR, -1);
Date previousYear = ca.getTime();
```
这段代码首先获取了当前时间的Calendar实例,然后将其设置为当前时间。接下来,使用`add`方法将年份减去1,最后通过`getTime`方法获取上一年的日期。
使用LocalDate类的示例代码如下:
```java
LocalDate currentDate = LocalDate.now();
LocalDate previousYear = currentDate.minusYears(1);
```
这段代码首先使用`LocalDate.now()`获取当前日期的实例,然后使用`minusYears`方法将年份减去1,最后得到上一年的日期。
无论是使用Calendar类还是LocalDate类,都可以很方便地获取当前时间的上一年。
#### 引用[.reference_title]
- *1* [Java获取当前时间的上一年、下一年、上个月、下个月、前一天等(时间格式化)](https://blog.csdn.net/qq_41563912/article/details/126284310)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^control_2,239^v3^insert_chatgpt"}} ] [.reference_item]
- *2* *3* [Java 获取日期前一年、月、日,指定日期的前几天,后几天](https://blog.csdn.net/m0_54790214/article/details/129280230)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^control_2,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
相关推荐
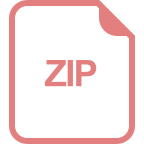
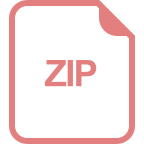












