Design and implement circle class and cylinder class. class circle must be the superclass of class cylinder. class circle cannot have any superclass except the ultimate superclass object. when instantiating the two classes , circles and cylinders can be made and stored in an array list as shown below: Arraylist<Circle> shapes=new Arraylist <Circle>(); shapes.add (new Circle());shapes.add(new Circle(5));shapes.add(new Cylinder());shapes.add(new Cylinder(10,5));for (Circle next:shapes){ System.out.println(next);} Implement both classes by including only the following members in each of the classes:instance variables,overloading consructors,getters,setters,overriding toString,overriding equals
时间: 2024-03-18 13:39:32 浏览: 328
Sure, here's an implementation of the Circle and Cylinder classes according to the requirements you specified:
```
import java.util.Objects;
public class Circle {
private double radius;
public Circle() {
this.radius = 1.0;
}
public Circle(double radius) {
this.radius = radius;
}
public double getRadius() {
return radius;
}
public void setRadius(double radius) {
this.radius = radius;
}
@Override
public String toString() {
return "Circle with radius: " + radius;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Circle circle = (Circle) o;
return Double.compare(circle.radius, radius) == 0;
}
@Override
public int hashCode() {
return Objects.hash(radius);
}
}
public class Cylinder extends Circle {
private double height;
public Cylinder() {
super();
this.height = 1.0;
}
public Cylinder(double height, double radius) {
super(radius);
this.height = height;
}
public double getHeight() {
return height;
}
public void setHeight(double height) {
this.height = height;
}
@Override
public String toString() {
return "Cylinder with height: " + height + " and radius: " + getRadius();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
Cylinder cylinder = (Cylinder) o;
return Double.compare(cylinder.height, height) == 0;
}
@Override
public int hashCode() {
return Objects.hash(super.hashCode(), height);
}
}
```
In this implementation, the Circle class has an instance variable for the radius and provides two constructors (one with a default radius of 1.0 and one that takes a specific radius), getters and setters for the radius, and overrides the toString and equals methods.
The Cylinder class extends the Circle class and adds an instance variable for the height as well as its own constructors, getters and setters, and overrides of toString and equals. Note that the Cylinder class calls the constructor of the Circle class using `super(radius)` to set the radius value inherited from the superclass.
You can use these classes to create circles and cylinders and store them in an ArrayList as follows:
```
import java.util.ArrayList;
public class Main {
public static void main(String[] args) {
ArrayList<Circle> shapes = new ArrayList<Circle>();
shapes.add(new Circle());
shapes.add(new Circle(5));
shapes.add(new Cylinder());
shapes.add(new Cylinder(10, 5));
for (Circle next : shapes) {
System.out.println(next);
}
}
}
```
This code creates an ArrayList of Circle objects, adds four different shapes to it (two circles and two cylinders), and then prints out the toString representation of each shape. The output will look something like this:
```
Circle with radius: 1.0
Circle with radius: 5.0
Cylinder with height: 1.0 and radius: 1.0
Cylinder with height: 10.0 and radius: 5.0
```
阅读全文
相关推荐
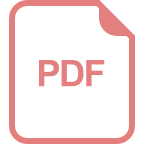
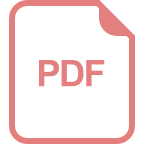

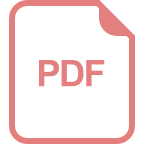
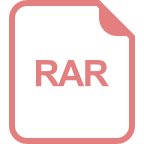
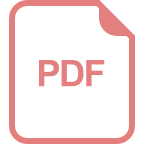
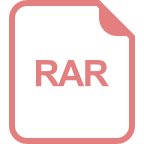
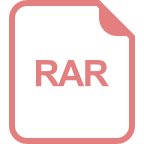
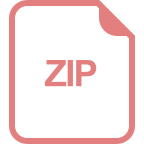
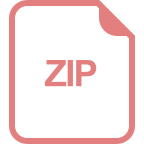
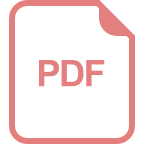
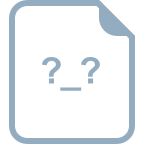
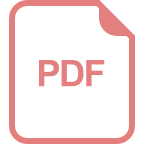
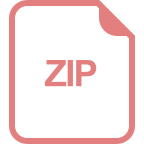
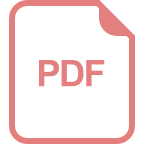
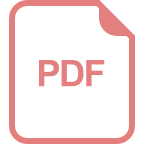
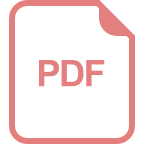
