使用Java语言实现单表操作共通方法。使用Mybatis和Springboot,数据库使用mysql,包括sql语句
时间: 2023-07-15 08:12:34 浏览: 179
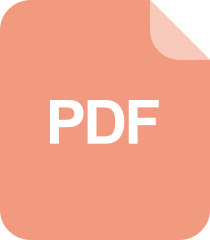
使用Mybatis对数据库进行单表操作的实现示例
下面是Java语言实现单表操作共通方法的示例代码,包含了Mybatis和Springboot的相关配置及实现:
1. 配置pom.xml文件,添加Mybatis和mysql的依赖:
```xml
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jdbc</artifactId>
</dependency>
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.1.3</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.23</version>
</dependency>
</dependencies>
```
2. 在application.properties文件中配置数据库连接信息:
```properties
spring.datasource.driverClassName=com.mysql.cj.jdbc.Driver
spring.datasource.url=jdbc:mysql://localhost:3306/test?useSSL=false&serverTimezone=UTC&allowPublicKeyRetrieval=true
spring.datasource.username=root
spring.datasource.password=root
```
3. 创建实体类,如下所示:
```java
public class User {
private Long id;
private String name;
private Integer age;
// getter and setter methods
}
```
4. 创建Mapper接口,定义CRUD方法,如下所示:
```java
@Mapper
public interface UserMapper {
@Insert("INSERT INTO user (name, age) VALUES (#{name}, #{age})")
@Options(useGeneratedKeys = true, keyProperty = "id")
int insert(User user);
@Update("UPDATE user SET name=#{name}, age=#{age} WHERE id=#{id}")
int update(User user);
@Delete("DELETE FROM user WHERE id=#{id}")
int delete(Long id);
@Select("SELECT * FROM user WHERE id=#{id}")
User selectById(Long id);
@Select("SELECT * FROM user")
List<User> selectAll();
}
```
5. 创建Service层,实现对Mapper接口的调用,并添加事务控制,如下所示:
```java
@Service
@Transactional
public class UserService {
@Autowired
private UserMapper userMapper;
public int insert(User user) {
return userMapper.insert(user);
}
public int update(User user) {
return userMapper.update(user);
}
public int delete(Long id) {
return userMapper.delete(id);
}
public User selectById(Long id) {
return userMapper.selectById(id);
}
public List<User> selectAll() {
return userMapper.selectAll();
}
}
```
6. 创建Controller层,暴露API接口,如下所示:
```java
@RestController
@RequestMapping("/user")
public class UserController {
@Autowired
private UserService userService;
@PostMapping("/insert")
public ApiResponse<User> insert(@RequestBody User user) {
userService.insert(user);
return ApiResponse.success(user);
}
@PostMapping("/update")
public ApiResponse<User> update(@RequestBody User user) {
userService.update(user);
return ApiResponse.success(user);
}
@PostMapping("/delete")
public ApiResponse<Long> delete(@RequestParam Long id) {
userService.delete(id);
return ApiResponse.success(id);
}
@GetMapping("/selectById")
public ApiResponse<User> selectById(@RequestParam Long id) {
User user = userService.selectById(id);
return ApiResponse.success(user);
}
@GetMapping("/selectAll")
public ApiResponse<List<User>> selectAll() {
List<User> users = userService.selectAll();
return ApiResponse.success(users);
}
}
```
7. 最后,定义ApiResponse类,用于封装API接口的返回结果,如下所示:
```java
public class ApiResponse<T> {
private Integer code;
private String message;
private T data;
public ApiResponse(Integer code, String message, T data) {
this.code = code;
this.message = message;
this.data = data;
}
public static <T> ApiResponse<T> success(T data) {
return new ApiResponse<>(200, "success", data);
}
// getter and setter methods
}
```
这样,就完成了Java语言实现单表操作共通方法的示例代码,包含了Mybatis和Springboot的相关配置及实现。
阅读全文
相关推荐
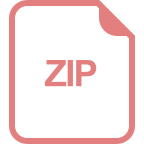
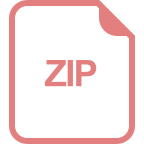















