3. 编写程序,要求如下 (1)接收用户从键盘输入的用户名和密码字符串 (2)对该密码字符串进行sha1()加密,打印输出 (3)将用户名和的加密后的密码存入password.txt文件 (4)关闭文件
时间: 2024-02-05 18:02:27 浏览: 76
好的,以下是Python 代码实现:
```python
import hashlib
# 接收用户输入的用户名和密码
username = input("请输入用户名:")
password = input("请输入密码:")
# 对密码字符串进行sha1加密
hash_object = hashlib.sha1(password.encode())
hex_dig = hash_object.hexdigest()
# 将用户名和加密后的密码存入文件
with open('password.txt', 'w') as f:
f.write(f"用户名:{username}\n")
f.write(f"加密后的密码:{hex_dig}")
# 关闭文件
f.close()
print("保存成功!")
```
请注意,此代码仅供学习参考,真实场景中,密码存储需考虑更多因素,如加盐等。
相关问题
. 编写程序,要求如下 (1)接收用户从键盘输入的用户名和密码字符串 (2)对该密码字符串进行sha1()加密,打印输出 (3)将用户名和的加密后的密码存入password.txt文件 (4)关闭文件
好的,我可以帮你实现这个程序。不过在开始之前,需要先安装Python内置的hashlib库,它提供了多种加密算法,包括SHA1加密算法。
以下是程序的代码实现:
```python
import hashlib
# 1. 接收用户输入的用户名和密码
username = input("请输入用户名:")
password = input("请输入密码:")
# 2. 对密码进行sha1()加密
sha1 = hashlib.sha1()
sha1.update(password.encode('utf-8')) # 将密码编码后进行加密
password_hash = sha1.hexdigest()
# 3. 将用户名和加密后的密码存入文件
with open('password.txt', 'a') as f:
f.write(username + ',' + password_hash + '\n')
# 4. 关闭文件
f.close()
print("用户名和密码已保存到password.txt文件中!")
```
程序运行时,会提示用户输入用户名和密码,然后对密码进行sha1()加密,并将用户名和加密后的密码存入一个名为password.txt的文件中,最后输出提示信息。
注意,程序中的密码是以明文形式接收的,但在存入文件前会进行加密处理。在实际应用中,应该采取更加安全的方式,如使用HTTPS协议进行数据传输,或使用更加安全的加密算法。
编写一个基于TCP协议的网络通信程序,一个客户程序,一个服务器程序。要求服务器通过socket连接后,接收客户端传来的用户名,判断为“liu”时,向客户端发送字符串“Hello,You are connected!”,否则,发送字符串“Sorry,you are not the user!”。客户端通过键盘输入用户名,发送到服务器,接收服务器发来的信息输出后结束。服务端显示客户端的IP地址。
好的,我会为您编写一个基于TCP协议的网络通信程序,分别包括客户端和服务器端。
服务端代码如下:
```python
import socket
HOST = '127.0.0.1'
PORT = 8000
def handle_request(client_socket):
buf = client_socket.recv(1024)
user = buf.decode()
if user == 'liu':
client_socket.send(b'Hello, You are connected!')
else:
client_socket.send(b'Sorry, you are not the user!')
print('Received from client:', user)
client_socket.close()
if __name__ == '__main__':
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_socket.bind((HOST, PORT))
server_socket.listen(1)
print('Server listening on port', PORT)
while True:
client_socket, client_addr = server_socket.accept()
print('Connected from', client_addr)
handle_request(client_socket)
```
客户端代码如下:
```python
import socket
HOST = '127.0.0.1'
PORT = 8000
if __name__ == '__main__':
client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
client_socket.connect((HOST, PORT))
user = input('Enter your username: ')
client_socket.send(user.encode())
response = client_socket.recv(1024)
print(response.decode())
client_socket.close()
```
使用方法:
1. 先运行服务端程序,命令行输入python server.py,服务端就会在本地8000端口监听客户端连接;
2. 再运行客户端程序,命令行输入python client.py,客户端会要求输入用户名,输入后按回车键发送给服务端;
3. 服务端接收到客户端发送的用户名后,判断是否为“liu”,根据判断结果发送不同的字符串给客户端;
4. 客户端接收到服务端发送的字符串后,打印输出,并关闭连接。
阅读全文
相关推荐
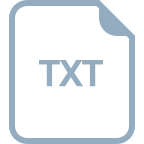
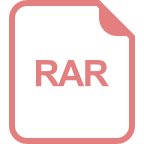
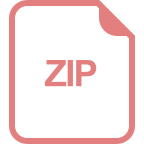
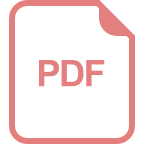
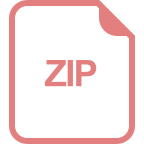
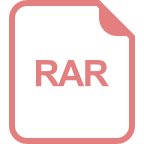
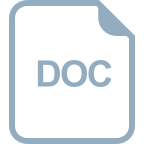
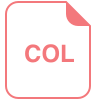
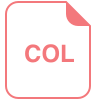
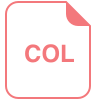
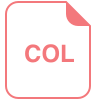
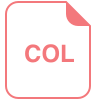
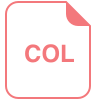
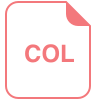
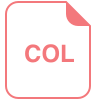