java excel导入数据库
时间: 2023-09-02 19:10:08 浏览: 55
以下是一个简单的Java代码示例,展示如何将Excel文件中的数据导入到数据库中:
1. 首先,你需要按照上面的方法读取Excel文件并获取单元格中的数据。
2. 然后,你需要建立一个连接到数据库的连接对象。
3. 接着,你需要使用PreparedStatement对象将数据插入到数据库中。
4. 最后,关闭连接对象和数据流。
下面是一个简单的Java代码示例,展示如何将Excel文件中的数据导入到数据库中:
```
import java.io.FileInputStream;
import java.io.IOException;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExcelImporter {
public static void main(String[] args) {
try {
FileInputStream file = new FileInputStream("path/to/excel/file.xlsx");
XSSFWorkbook workbook = new XSSFWorkbook(file);
// 获取第一个工作表
XSSFSheet sheet = workbook.getSheetAt(0);
// 建立数据库连接
Class.forName("com.mysql.jdbc.Driver");
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password");
// 插入数据
String sql = "INSERT INTO mytable (column1, column2, column3) VALUES (?, ?, ?)";
PreparedStatement statement = conn.prepareStatement(sql);
for (Row row : sheet) {
Cell cell1 = row.getCell(0);
Cell cell2 = row.getCell(1);
Cell cell3 = row.getCell(2);
statement.setString(1, cell1.getStringCellValue());
statement.setInt(2, (int) cell2.getNumericCellValue());
statement.setDouble(3, cell3.getNumericCellValue());
statement.executeUpdate();
}
statement.close();
conn.close();
file.close();
} catch (IOException | ClassNotFoundException | SQLException e) {
e.printStackTrace();
}
}
}
```
当然,你也可以根据自己的需求对代码进行修改。
相关推荐









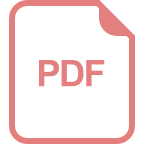
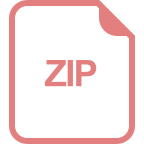