java将excel导入数据库
时间: 2024-05-06 13:17:29 浏览: 6
可以使用Java中的Apache POI来读取Excel文件,并结合JDBC连接数据库将数据导入到数据库中。
以下是一个简单的示例代码,假设你要将Excel中的数据导入到名为"table_name"的数据库表中:
```java
import java.io.FileInputStream;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExcelToDatabase {
public static void main(String[] args) {
String jdbcURL = "jdbc:mysql://localhost:3306/database_name";
String username = "username";
String password = "password";
String excelFilePath = "path/to/excel/file.xlsx";
try (Connection connection = DriverManager.getConnection(jdbcURL, username, password);
FileInputStream inputStream = new FileInputStream(excelFilePath);
XSSFWorkbook workbook = new XSSFWorkbook(inputStream)) {
Sheet sheet = workbook.getSheetAt(0);
String sql = "INSERT INTO table_name (column1, column2, column3) VALUES (?, ?, ?)";
PreparedStatement statement = connection.prepareStatement(sql);
for (int i = 1; i <= sheet.getLastRowNum(); i++) {
Row currentRow = sheet.getRow(i);
Cell cell1 = currentRow.getCell(0);
statement.setString(1, cell1.getStringCellValue());
Cell cell2 = currentRow.getCell(1);
statement.setString(2, cell2.getStringCellValue());
Cell cell3 = currentRow.getCell(2);
statement.setString(3, cell3.getStringCellValue());
statement.executeUpdate();
}
System.out.println("Data imported successfully");
} catch (SQLException e) {
System.out.println("Database error: " + e.getMessage());
} catch (Exception e) {
System.out.println("Error: " + e.getMessage());
}
}
}
```
请替换代码中的数据库连接信息、Excel文件路径、表名和列名等信息,以适应你的情况。注意,代码中默认将Excel文件的第一行视为表头,因此从第二行开始读取数据。
另外,需要在项目中引入Apache POI和MySQL JDBC驱动的依赖。
相关推荐
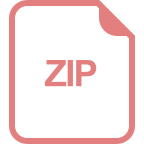









